tkinter socket
时间: 2023-09-12 16:07:03 浏览: 114
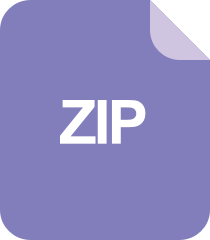
Python实现的多人聊天室源码,基于socket tcp通信,使用tkinter做客户端界面,含可执行文件

Tkinter is a standard Python library for creating graphical user interfaces (GUIs). It provides a set of widgets and tools for building desktop applications. On the other hand, sockets are a low-level networking interface in Python used for communication between two machines over a network.
To use sockets with Tkinter, you can create a GUI application that sends or receives data over a network using sockets. For example, you can create a chat application that allows users to send and receive messages over a network using sockets.
Here's an example code snippet that demonstrates how to create a simple chat application using Tkinter and sockets:
```python
import socket
import tkinter as tk
# Create a socket object
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Connect to the server
server_address = ('localhost', 10000)
sock.connect(server_address)
# Create the GUI
root = tk.Tk()
root.title("Chat")
# Create the input box and send button
input_box = tk.Entry(root)
input_box.pack(side=tk.LEFT)
send_button = tk.Button(root, text="Send")
# Define the function to send data
def send_data():
message = input_box.get()
sock.sendall(message.encode('utf-8'))
input_box.delete(0, tk.END)
# Bind the send button to the send_data function
send_button.config(command=send_data)
send_button.pack(side=tk.RIGHT)
# Start the main loop
while True:
# Receive data from the server
data = sock.recv(1024).decode('utf-8')
# Display the data in the GUI
if data:
tk.Label(root, text=data).pack()
# Update the GUI
root.update()
```
In this example, we first create a socket object and connect to a server at the specified address. Then we create a GUI using Tkinter and define an input box and a send button. We also define a `send_data` function that sends the data entered in the input box to the server using the socket object.
Finally, we start a loop that receives data from the server using the socket object and displays it in the GUI. We also update the GUI using the `root.update()` method.
阅读全文
相关推荐
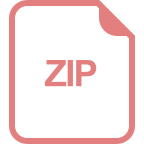
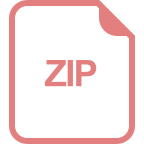















