C++生成comtrade格式数据的代码
时间: 2023-11-28 10:04:26 浏览: 216
以下是一个 C++ 的示例代码,可以生成符合 COMTRADE 格式的数据文件。您可以根据自己的需求进行修改。
```cpp
#include <iostream>
#include <fstream>
#include <ctime>
#include <cstring>
#include <cmath>
using namespace std;
const int MAX_FILENAME_LEN = 256;
const int MAX_CHANNEL_COUNT = 16;
struct ConfigData {
float sampling_rate;
char date_time[24];
char version[8];
char station_name[32];
char rec_dev_id[16];
char cfg_dev_id[16];
int analog_channel_count;
int digital_channel_count;
char analog_channel_names[MAX_CHANNEL_COUNT][16];
char digital_channel_names[MAX_CHANNEL_COUNT][16];
};
int main() {
// 定义文件名和文件路径
char filename[MAX_FILENAME_LEN] = "example";
char filepath[MAX_FILENAME_LEN] = "/path/to/save";
// 定义数据参数
ConfigData config = {
8000.0f,
"2022-01-01 00:00:00.000",
"1999a",
"My Station",
"My Device",
"My Device",
2,
4,
{"V1", "I1"},
{"Status1", "Status2", "Status3", "Status4"}
};
int sample_count = 8000;
float analog_data[sample_count][MAX_CHANNEL_COUNT] = {0};
int digital_data[sample_count][MAX_CHANNEL_COUNT] = {0};
// 写入cfg文件
char cfg_filename[MAX_FILENAME_LEN];
snprintf(cfg_filename, MAX_FILENAME_LEN, "%s/%s.cfg", filepath, filename);
ofstream cfg_file(cfg_filename);
cfg_file << "FREQ " << config.sampling_rate << endl;
cfg_file << "DATE " << config.date_time << endl;
cfg_file << "VERSION " << config.version << endl;
cfg_file << "STATION_NAME " << config.station_name << endl;
cfg_file << "REC_DEV_ID " << config.rec_dev_id << endl;
cfg_file << "CFG_DEV_ID " << config.cfg_dev_id << endl;
cfg_file << "NUM_ANALOG " << config.analog_channel_count << endl;
cfg_file << "NUM_DIGITAL " << config.digital_channel_count << endl;
for (int i = 0; i < config.analog_channel_count; i++) {
cfg_file << "ANALOG_CHAN " << i+1 << " " << config.analog_channel_names[i] << endl;
}
for (int i = 0; i < config.digital_channel_count; i++) {
cfg_file << "DIGITAL_CHAN " << i+1 << " " << config.digital_channel_names[i] << endl;
}
cfg_file << "END" << endl;
cfg_file.close();
// 写入dat文件
char dat_filename[MAX_FILENAME_LEN];
snprintf(dat_filename, MAX_FILENAME_LEN, "%s/%s.dat", filepath, filename);
ofstream dat_file(dat_filename, ios::binary);
for (int i = 0; i < sample_count; i++) {
for (int j = 0; j < config.analog_channel_count; j++) {
dat_file.write(reinterpret_cast<char*>(&analog_data[i][j]), sizeof(float));
}
for (int j = 0; j < config.digital_channel_count; j++) {
dat_file.write(reinterpret_cast<char*>(&digital_data[i][j]), sizeof(int));
}
}
dat_file.close();
return 0;
}
```
以上代码生成了一个符合 COMTRADE 格式的数据文件,包括 .cfg 和 .dat 两个文件。您需要修改代码中的参数来生成不同的数据文件。
阅读全文
相关推荐
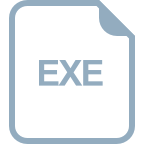









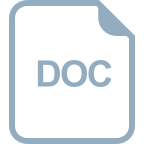






