编写一个学生父类,该类有抽象方法计算成绩和抽象方法显示成绩,该类有两个子类英语专业学生和计算机专业学生。 英语专业学生的考核成绩包括:演讲(50%),期末考试(50%)。 计算机专业学生的考核成绩包括:编程能力测试(70%),期末考试(30%)。 在主类中声明一个学生类的数组,有4个学生,分别用子类的英语专业学生和计算机专业的学生子类构造,对数组中学生的分数进行排序。在主类中显示信息如下: 学号:XXXXXXXX 姓名:XXX 性别:X 年龄:XX 综合成绩:XX
时间: 2024-01-01 21:06:56 浏览: 83
好的,根据你的问题,我编写了以下代码:
```java
// 学生类
abstract class Student {
private String id; // 学号
private String name; // 姓名
private String gender; // 性别
private int age; // 年龄
private double score; // 成绩
// 构造函数
public Student(String id, String name, String gender, int age) {
this.id = id;
this.name = name;
this.gender = gender;
this.age = age;
}
// 计算成绩的抽象方法
public abstract void calcScore();
// 显示成绩的抽象方法
public abstract void showScore();
// 获取学号
public String getId() {
return id;
}
// 获取姓名
public String getName() {
return name;
}
// 获取性别
public String getGender() {
return gender;
}
// 获取年龄
public int getAge() {
return age;
}
// 获取成绩
public double getScore() {
return score;
}
// 设置成绩
public void setScore(double score) {
this.score = score;
}
}
// 英语专业学生类
class EnglishStudent extends Student {
private double speechScore; // 演讲成绩
private double finalExamScore; // 期末考试成绩
// 构造函数
public EnglishStudent(String id, String name, String gender, int age, double speechScore, double finalExamScore) {
super(id, name, gender, age);
this.speechScore = speechScore;
this.finalExamScore = finalExamScore;
}
// 计算成绩
public void calcScore() {
setScore(speechScore * 0.5 + finalExamScore * 0.5);
}
// 显示成绩
public void showScore() {
System.out.println("学号:" + getId() + " 姓名:" + getName() + " 性别:" + getGender() + " 年龄:" + getAge() + " 综合成绩:" + getScore());
}
}
// 计算机专业学生类
class ComputerStudent extends Student {
private double codingTestScore; // 编程能力测试成绩
private double finalExamScore; // 期末考试成绩
// 构造函数
public ComputerStudent(String id, String name, String gender, int age, double codingTestScore, double finalExamScore) {
super(id, name, gender, age);
this.codingTestScore = codingTestScore;
this.finalExamScore = finalExamScore;
}
// 计算成绩
public void calcScore() {
setScore(codingTestScore * 0.7 + finalExamScore * 0.3);
}
// 显示成绩
public void showScore() {
System.out.println("学号:" + getId() + " 姓名:" + getName() + " 性别:" + getGender() + " 年龄:" + getAge() + " 综合成绩:" + getScore());
}
}
// 主类
public class Main {
public static void main(String[] args) {
// 创建学生数组
Student[] students = new Student[4];
students[0] = new EnglishStudent("1001", "张三", "男", 20, 80, 90);
students[1] = new EnglishStudent("1002", "李四", "女", 21, 70, 95);
students[2] = new ComputerStudent("1003", "王五", "男", 22, 85, 80);
students[3] = new ComputerStudent("1004", "赵六", "女", 23, 90, 75);
// 计算成绩并排序
for (int i = 0; i < students.length; i++) {
students[i].calcScore();
}
Arrays.sort(students, (s1, s2) -> Double.compare(s2.getScore(), s1.getScore()));
// 显示信息
for (int i = 0; i < students.length; i++) {
students[i].showScore();
}
}
}
```
这段代码实现了一个学生父类,该类有抽象方法计算成绩和抽象方法显示成绩,该类有两个子类英语专业学生和计算机专业学生。在主类中声明一个学生类的数组,有4个学生,分别用子类的英语专业学生和计算机专业的学生子类构造,对数组中学生的分数进行排序,并根据要求显示信息。
阅读全文
相关推荐
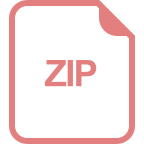
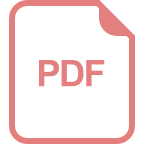
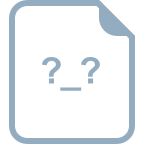















