用Android studio通过java语言写一个坦克大战游戏
时间: 2023-12-21 16:05:52 浏览: 179
好的,下面是一个简单的坦克大战游戏的实现思路,代码中可能不完整,请根据自己的需求进行修改。
1. 创建一个基本的 Android 工程,添加游戏所需的资源文件,如游戏背景、坦克图片、子弹图片等。
2. 创建一个 Tank 类,表示游戏中的坦克。Tank 类包含坦克的位置、方向、速度、是否存活等属性,以及坦克的移动、开火等方法。
3. 创建一个 Bullet 类,表示游戏中的子弹。Bullet 类包含子弹的位置、方向、速度等属性,以及子弹的移动、碰撞检测等方法。
4. 创建一个 GameView 类,表示游戏的主界面。GameView 中包含一个 Tank 对象和多个 Bullet 对象,以及游戏的主循环,负责更新 Tank 和 Bullet 的位置、处理用户输入、检测碰撞等逻辑。
5. 在 MainActivity 中创建一个 GameView 实例,并将其设置为 ContentView。
下面是一份参考代码:
Tank 类:
```
public class Tank {
private Bitmap bitmap;
private int x, y;
private int direction;
private int speed;
private boolean isAlive;
public Tank(Bitmap bitmap, int x, int y) {
this.bitmap = bitmap;
this.x = x;
this.y = y;
this.direction = 0;
this.speed = 5;
this.isAlive = true;
}
public void move() {
switch (direction) {
case 0: // 向上
y -= speed;
break;
case 1: // 向右
x += speed;
break;
case 2: // 向下
y += speed;
break;
case 3: // 向左
x -= speed;
break;
}
}
public void fire(List<Bullet> bullets) {
bullets.add(new Bullet(x, y, direction));
}
// 碰撞检测
public boolean isCollideWith(Bullet bullet) {
Rect rect1 = new Rect(x - bitmap.getWidth() / 2,
y - bitmap.getHeight() / 2,
x + bitmap.getWidth() / 2,
y + bitmap.getHeight() / 2);
Rect rect2 = new Rect(bullet.getX() - bullet.getBitmap().getWidth() / 2,
bullet.getY() - bullet.getBitmap().getHeight() / 2,
bullet.getX() + bullet.getBitmap().getWidth() / 2,
bullet.getY() + bullet.getBitmap().getHeight() / 2);
return rect1.intersect(rect2);
}
// getter 和 setter
}
```
Bullet 类:
```
public class Bullet {
private Bitmap bitmap;
private int x, y;
private int direction;
private int speed;
public Bullet(int x, int y, int direction) {
this.bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.bullet);
this.x = x;
this.y = y;
this.direction = direction;
this.speed = 10;
}
public void move() {
switch (direction) {
case 0: // 向上
y -= speed;
break;
case 1: // 向右
x += speed;
break;
case 2: // 向下
y += speed;
break;
case 3: // 向左
x -= speed;
break;
}
}
// getter 和 setter
}
```
GameView 类:
```
public class GameView extends SurfaceView implements SurfaceHolder.Callback {
private Tank tank;
private List<Bullet> bullets;
private boolean isRunning;
public GameView(Context context) {
super(context);
getHolder().addCallback(this);
tank = new Tank(BitmapFactory.decodeResource(getResources(), R.drawable.tank), 300, 500);
bullets = new ArrayList<>();
isRunning = true;
}
@Override
public void surfaceCreated(SurfaceHolder holder) {
new Thread(new Runnable() {
@Override
public void run() {
while (isRunning) {
// 更新坦克位置
tank.move();
// 更新子弹位置
for (Bullet bullet : bullets) {
bullet.move();
}
// 绘制游戏界面
draw();
// 处理用户输入
handleInput();
// 检测碰撞
checkCollide();
}
}
}).start();
}
private void draw() {
Canvas canvas = getHolder().lockCanvas();
if (canvas != null) {
canvas.drawColor(Color.WHITE); // 清空画布
canvas.drawBitmap(tank.getBitmap(), tank.getX() - tank.getBitmap().getWidth() / 2,
tank.getY() - tank.getBitmap().getHeight() / 2, null); // 绘制坦克
for (Bullet bullet : bullets) {
canvas.drawBitmap(bullet.getBitmap(), bullet.getX() - bullet.getBitmap().getWidth() / 2,
bullet.getY() - bullet.getBitmap().getHeight() / 2, null); // 绘制子弹
}
getHolder().unlockCanvasAndPost(canvas);
}
}
private void handleInput() {
// 处理用户输入,例如按键事件等
}
private void checkCollide() {
// 检测坦克和子弹的碰撞
for (Bullet bullet : bullets) {
if (tank.isCollideWith(bullet)) {
tank.setAlive(false);
bullet.setAlive(false);
}
}
// 移除已经死亡的子弹
Iterator<Bullet> iterator = bullets.iterator();
while (iterator.hasNext()) {
Bullet bullet = iterator.next();
if (!bullet.isAlive()) {
iterator.remove();
}
}
}
@Override
public void surfaceChanged(SurfaceHolder holder, int format, int width, int height) {
}
@Override
public void surfaceDestroyed(SurfaceHolder holder) {
isRunning = false;
}
}
```
MainActivity 类:
```
public class MainActivity extends AppCompatActivity {
private GameView gameView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
gameView = new GameView(this);
setContentView(gameView);
}
}
```
这样一个简单的坦克大战游戏就完成了。当然,这只是一个基本的框架,你还需要根据自己的需求添加更多的功能,例如敌方坦克、道具等。
阅读全文
相关推荐





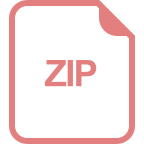

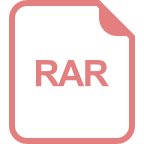




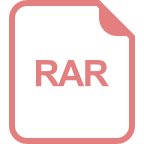

