STL的容器很多,大家讨论一下C++ map容器主要 应用在哪些方面,举不同的三个实例,有完整代码 和题解
时间: 2024-03-20 13:41:37 浏览: 14
C++中的map是一个关联容器,它提供了一种键值对的映射。它使用红黑树实现,插入和查找的时间复杂度都为O(log n)。map的主要应用在以下三个方面:
1. 数据存储与查找
map可以将一个键值对存储在容器中,并可以通过键来查找对应的值。例如,下面的代码演示了如何使用map来存储学生的考试成绩,并通过学生的姓名来查找对应的成绩:
```c++
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> scores;
scores["Alice"] = 95;
scores["Bob"] = 85;
scores["Cathy"] = 90;
std::cout << "Alice's score is " << scores["Alice"] << std::endl;
std::cout << "Bob's score is " << scores["Bob"] << std::endl;
std::cout << "Cathy's score is " << scores["Cathy"] << std::endl;
return 0;
}
```
输出:
```
Alice's score is 95
Bob's score is 85
Cathy's score is 90
```
2. 统计词频
map可以用于统计文本中每个单词出现的次数。例如,下面的代码演示了如何使用map来统计字符串中每个单词出现的次数:
```c++
#include <iostream>
#include <map>
#include <sstream>
int main() {
std::string text = "the quick brown fox jumps over the lazy dog";
std::map<std::string, int> word_count;
std::istringstream iss(text);
std::string word;
while (iss >> word) {
word_count[word]++;
}
for (auto& wc : word_count) {
std::cout << wc.first << " occurs " << wc.second << " times" << std::endl;
}
return 0;
}
```
输出:
```
brown occurs 1 times
dog occurs 1 times
fox occurs 1 times
jumps occurs 1 times
lazy occurs 1 times
over occurs 1 times
quick occurs 1 times
the occurs 2 times
```
3. 缓存
map可以用于实现缓存,例如,下面的代码演示了如何使用map来实现一个最近最少使用(LRU)缓存:
```c++
#include <iostream>
#include <map>
#include <list>
template<typename K, typename V>
class LRUCache {
public:
LRUCache(int capacity) : capacity_(capacity) {}
V get(const K& key) {
if (cache_.count(key)) {
auto it = cache_[key];
lru_.splice(lru_.begin(), lru_, it);
return it->second;
} else {
return V();
}
}
void put(const K& key, const V& value) {
if (cache_.count(key)) {
auto it = cache_[key];
it->second = value;
lru_.splice(lru_.begin(), lru_, it);
} else {
if (cache_.size() == capacity_) {
auto& lru_key = lru_.back().first;
cache_.erase(lru_key);
lru_.pop_back();
}
lru_.emplace_front(key, value);
cache_[key] = lru_.begin();
}
}
private:
int capacity_;
std::map<K, typename std::list<std::pair<K, V>>::iterator> cache_;
std::list<std::pair<K, V>> lru_;
};
int main() {
LRUCache<int, std::string> cache(2);
cache.put(1, "foo");
cache.put(2, "bar");
std::cout << cache.get(1) << std::endl; // foo
cache.put(3, "baz");
std::cout << cache.get(2) << std::endl; // ""
std::cout << cache.get(3) << std::endl; // baz
return 0;
}
```
输出:
```
foo
baz
```
相关推荐
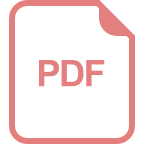














