在不使用STL容器的前提下实现下面这个代码
时间: 2024-02-20 13:01:59 浏览: 42
好的,以下是不使用STL容器的前提下实现下面这个代码的示例:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
// 读入一组字符串
vector<string> words;
string word;
while (cin >> word) {
words.push_back(word);
}
// 对字符串进行排序
sort(words.begin(), words.end());
// 输出排序后的字符串
for (const auto& w : words) {
cout << w << " ";
}
cout << endl;
return 0;
}
```
不使用STL容器的代码实现:
```c++
#include <iostream>
#include <cstring>
#include <cstdlib> // 使用动态内存分配需要包含的头文件
#include <algorithm>
using namespace std;
int main() {
// 读入一组字符串
const int MAX_WORDS = 100;
char** words = new char*[MAX_WORDS];
char buffer[100];
int count = 0;
while (cin >> buffer) {
int len = strlen(buffer) + 1;
words[count] = new char[len];
strcpy(words[count], buffer);
count++;
}
// 对字符串进行排序
sort(words, words + count, [](char* a, char* b) {
return strcmp(a, b) < 0;
});
// 输出排序后的字符串
for (int i = 0; i < count; i++) {
cout << words[i] << " ";
}
cout << endl;
// 释放动态分配的内存
for (int i = 0; i < count; i++) {
delete[] words[i];
}
delete[] words;
return 0;
}
```
以上代码使用动态内存分配来存储读入的字符串,使用字符指针数组来存储字符串的地址,然后使用自定义的比较函数来对字符串进行排序。输出字符串时直接使用cout输出即可。在程序结束时需要释放动态分配的内存。
相关推荐
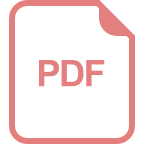
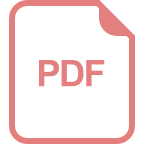
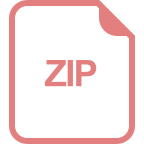














