用 vue实现3d模型显示
时间: 2024-04-29 08:23:33 浏览: 11
要使用 Vue 实现 3D 模型显示,可以使用 Three.js 库。Three.js 是一个用于创建和渲染 3D 图形的 JavaScript 库,它可以与 Vue 框架集成。
以下是一个简单的示例,演示如何在 Vue 中使用 Three.js 显示 3D 模型:
1.在 Vue 项目中安装 Three.js 库:
```
npm install three --save
```
2.创建一个 Vue 组件,该组件将显示 3D 模型:
```
<template>
<div ref="container"></div>
</template>
<script>
import * as THREE from 'three';
export default {
mounted() {
// 创建 Three.js 场景和相机
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建 Three.js 渲染器并将其添加到 HTML 元素中
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
this.$refs.container.appendChild(renderer.domElement);
// 创建 Three.js 几何体和材质,并将它们添加到场景中
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
// 设置相机位置和方向
camera.position.z = 5;
// 渲染场景
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
},
};
</script>
```
在上面的代码中,我们创建了一个 Vue 组件,其中的 `mounted` 钩子函数用于初始化 Three.js 场景、相机、渲染器、几何体和材质,并将它们添加到场景中。然后,我们设置相机位置和方向,并使用 `requestAnimationFrame` 函数来渲染场景。在 `animate` 函数中,我们旋转了几何体,以便可以看到其 3D 效果。
3.在 Vue 应用程序中使用该组件:
```
<template>
<div>
<my-3d-model />
</div>
</template>
<script>
import My3DModel from './My3DModel.vue';
export default {
components: {
My3DModel,
},
};
</script>
```
在上面的代码中,我们在 Vue 应用程序中使用了 `My3DModel` 组件,它将显示 3D 模型。
这是一个简单的示例,演示了如何在 Vue 中使用 Three.js 显示 3D 模型。您可以使用 Three.js 库的其他功能来创建更复杂的 3D 场景和模型。
相关推荐
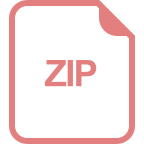
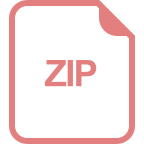














