定义一boat与car两个类,二者都有weight属性,定义二者的一个友元函数totalweight(),计算二者的重量和
时间: 2024-05-11 15:15:15 浏览: 20
好的,这是一个关于C++编程的问题。boat和car是两个类,都有weight属性,我们可以在类中定义这个属性。然后定义一个友元函数totalweight(),在这个函数中,我们可以访问boat和car的weight属性并计算它们的总重量。代码如下所示:
```
class boat {
friend float totalweight(boat b, car c);
private:
float weight;
public:
boat(float w) {
weight = w;
}
};
class car {
friend float totalweight(boat b, car c);
private:
float weight;
public:
car(float w) {
weight = w;
}
};
float totalweight(boat b, car c) {
return b.weight + c.weight;
}
```
调用示例:
```
boat b(100);
car c(200);
float total = totalweight(b, c);
```
这个程序将输出300.
相关问题
定义Boat和Car两个类,二者都有weight属性,()定义二者的一个友元函数get total weight
假设 Boat 和 Car 类的定义如下:
```cpp
class Boat {
private:
int weight;
public:
Boat(int w) : weight(w) {}
// other member functions
friend int getTotalWeight(const Boat& b, const Car& c);
};
class Car {
private:
int weight;
public:
Car(int w) : weight(w) {}
// other member functions
friend int getTotalWeight(const Boat& b, const Car& c);
};
```
可以看到,我们在类的定义中声明了一个友元函数 `getTotalWeight`,该函数的参数为一个 `Boat` 对象和一个 `Car` 对象。由于该函数是友元函数,因此可以访问 `Boat` 和 `Car` 类的私有成员变量。
下面是 `getTotalWeight` 函数的实现:
```cpp
int getTotalWeight(const Boat& b, const Car& c) {
return b.weight + c.weight;
}
```
该函数返回 `Boat` 和 `Car` 对象的重量之和。
使用示例:
```cpp
int main() {
Boat b(100);
Car c(200);
int totalWeight = getTotalWeight(b, c);
cout << "Total weight: " << totalWeight << endl; // 输出 Total weight: 300
return 0;
}
```
定义Boat与Car两个类,二者都有weight属性,定义二者的一个友元函数totalWeight(),计算二者的重量和。
以下是C++代码实现:
```cpp
#include <iostream>
using namespace std;
class Car; // 前置声明
class Boat {
private:
int weight;
public:
Boat(int w) : weight(w) {}
friend int totalWeight(Boat& b, Car& c); // 声明友元函数
};
class Car {
private:
int weight;
public:
Car(int w) : weight(w) {}
friend int totalWeight(Boat& b, Car& c); // 声明友元函数
};
int totalWeight(Boat& b, Car& c) { // 友元函数实现
return b.weight + c.weight;
}
int main() {
Boat b(100);
Car c(200);
cout << "Total weight: " << totalWeight(b, c) << endl; // 输出 Total weight: 300
return 0;
}
```
在上述代码中,我们定义了两个类 `Boat` 和 `Car`,分别有一个私有成员变量 `weight`。为了能够访问对方的私有成员变量,我们定义了一个名为 `totalWeight` 的友元函数,该函数可以访问 `Boat` 和 `Car` 类的私有成员变量。在 `main` 函数中,我们创建了一个 `Boat` 对象和一个 `Car` 对象,然后调用 `totalWeight` 函数计算二者的重量和,并输出结果。
相关推荐
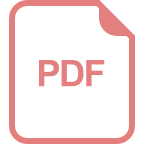
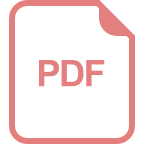











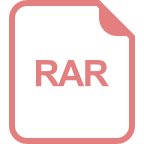