请用c语言写一个查询曲库内歌曲的函数
时间: 2023-11-28 16:48:45 浏览: 25
好的,以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
// 定义歌曲结构体
typedef struct {
char name[50]; // 歌曲名称
char artist[50]; // 歌手
int year; // 发行年份
} Song;
// 假设有以下几首歌曲
Song songs[] = {
{"Shape of You", "Ed Sheeran", 2017},
{"Stressed Out", "Twenty One Pilots", 2015},
{"Counting Stars", "OneRepublic", 2013},
{"Uptown Funk", "Mark Ronson ft. Bruno Mars", 2014},
{"All of Me", "John Legend", 2013},
{"Love Story", "Taylor Swift", 2008},
{"Roar", "Katy Perry", 2013},
{"Someone Like You", "Adele", 2011},
{"Hello", "Adele", 2015},
{"Radioactive", "Imagine Dragons", 2012}
};
// 查询歌曲函数
void searchSong(char *query) {
int i, found = 0;
for (i = 0; i < sizeof(songs) / sizeof(Song); i++) {
if (strstr(songs[i].name, query) != NULL) {
printf("%s - %s (%d)\n", songs[i].artist, songs[i].name, songs[i].year);
found = 1;
}
}
if (!found) {
printf("No results found.\n");
}
}
int main() {
// 搜索歌曲
searchSong("Love");
searchSong("Bruno Mars");
searchSong("2015");
searchSong("Justin Bieber");
return 0;
}
```
该代码定义了一个 `Song` 结构体,包含了歌曲名称、歌手和发行年份等信息,然后定义了一个 `songs` 数组,用于存储多首歌曲的信息。`searchSong` 函数接收一个字符串参数 `query`,用于作为查询关键词,遍历 `songs` 数组,查找包含该关键词的歌曲,并输出歌曲的详细信息。如果没有找到符合条件的歌曲,则输出提示信息。
在主函数中,我们可以多次调用 `searchSong` 函数,传入不同的查询关键词进行测试。
相关推荐
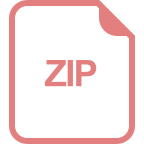
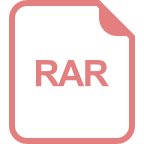
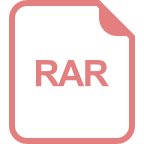














