用python图书管理系统网页
时间: 2023-09-14 18:06:27 浏览: 49
要开发一个图书管理系统网页,您可以使用Python编程语言和Web框架(如Flask或Django)。以下是简单的步骤:
1. 安装Python和所选的Web框架。
2. 定义模型类来表示图书,包括属性如书名、作者、出版日期等。您可以使用ORM(对象关系映射)库,如SQLAlchemy。
3. 创建视图函数来处理HTTP请求,例如添加、更新、删除和检索图书的请求。您可以使用模板引擎(如Jinja2)来渲染HTML模板。
4. 创建URL路由来将HTTP请求映射到相应的视图函数。
5. 建立数据库连接并初始化数据表。
6. 运行应用程序并访问网站。
以下是基于Flask框架的示例代码:
```python
from flask import Flask, render_template, request, redirect, url_for
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///books.db'
db = SQLAlchemy(app)
class Book(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(80), nullable=False)
author = db.Column(db.String(50), nullable=False)
published_date = db.Column(db.Date, nullable=False)
description = db.Column(db.Text)
@app.route('/')
def index():
books = Book.query.all()
return render_template('index.html', books=books)
@app.route('/add', methods=['GET', 'POST'])
def add_book():
if request.method == 'POST':
title = request.form['title']
author = request.form['author']
published_date = request.form['published_date']
description = request.form['description']
book = Book(title=title, author=author, published_date=published_date, description=description)
db.session.add(book)
db.session.commit()
return redirect(url_for('index'))
else:
return render_template('add_book.html')
@app.route('/edit/<int:id>', methods=['GET', 'POST'])
def edit_book(id):
book = Book.query.get_or_404(id)
if request.method == 'POST':
book.title = request.form['title']
book.author = request.form['author']
book.published_date = request.form['published_date']
book.description = request.form['description']
db.session.commit()
return redirect(url_for('index'))
else:
return render_template('edit_book.html', book=book)
@app.route('/delete/<int:id>', methods=['POST'])
def delete_book(id):
book = Book.query.get_or_404(id)
db.session.delete(book)
db.session.commit()
return redirect(url_for('index'))
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
```
在这个示例中,我们使用Flask和SQLAlchemy来创建了一个基本的图书管理系统网页。我们定义了一个`Book`模型类来表示图书,并使用Flask路由来处理HTTP请求。
在这个示例中,我们使用了SQLite数据库,但您可以使用其他数据库,如MySQL或PostgreSQL。
您还需要创建HTML模板来呈现网页。在这个示例中,我们使用了Jinja2模板引擎来渲染模板。以下是`index.html`模板的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>My Library</title>
</head>
<body>
<h1>My Library</h1>
<table>
<thead>
<tr>
<th>Title</th>
<th>Author</th>
<th>Published Date</th>
<th>Description</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
{% for book in books %}
<tr>
<td>{{ book.title }}</td>
<td>{{ book.author }}</td>
<td>{{ book.published_date }}</td>
<td>{{ book.description }}</td>
<td>
<a href="{{ url_for('edit_book', id=book.id) }}">Edit</a>
<form action="{{ url_for('delete_book', id=book.id) }}" method="post">
<input type="submit" value="Delete">
</form>
</td>
</tr>
{% endfor %}
</tbody>
</table>
<a href="{{ url_for('add_book') }}">Add Book</a>
</body>
</html>
```
这个模板使用了一个`for`循环来显示所有图书,并且包含添加、编辑和删除按钮。您需要创建其他模板来处理这些操作。
这只是一个简单的示例,您可以根据您的需求进行修改和扩展。
相关推荐
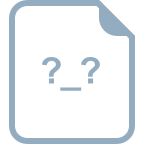
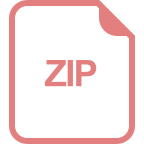
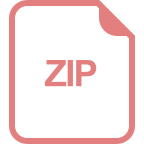














