母亲节是每年的五月第二个星期天,针对输入的年份,计算该年的哪一天是母亲节。C语言实现
时间: 2024-05-14 20:19:05 浏览: 119
以下是C语言实现代码:
```c
#include <stdio.h>
int main() {
int year, day;
printf("请输入年份:");
scanf("%d", &year);
day = 14 - ((5 + year / 4 - year / 100 + year / 400) % 7);
if (day <= 0) {
day += 7;
}
printf("%d年的母亲节是%d月%d日\n", year, 5, day);
return 0;
}
```
该程序通过公式计算出每年的母亲节是哪一天,然后输出结果。其中,公式为:
```
day = 14 - ((5 + year / 4 - year / 100 + year / 400) % 7);
```
该公式中,year为输入的年份,day为计算出的母亲节是哪一天。公式的计算过程为:
1. 计算出该年5月1日是星期几,公式为:`(5 + year / 4 - year / 100 + year / 400) % 7`。
2. 如果5月1日是星期日,则母亲节是5月的第二个星期日,即14号;如果5月1日不是星期日,则母亲节是5月的第一个星期日,即14减去5月1日是星期几的天数。
3. 如果计算出的天数小于等于0,则母亲节应该是上一周的星期日,即加上7天。
最后输出结果时,月份固定为5月。
相关问题
母亲节是每年的五月第二个星期天,针对输入的年份,计算该年的哪一天是母亲节。,用C语言编程
以下是用C语言编写的计算母亲节日期的程序:
```c
#include <stdio.h>
int main() {
int year, day, month;
printf("请输入年份:");
scanf("%d", &year);
// 计算母亲节的日期
day = 14 - (1 + 5 * ((year / 4) - (year / 100) + (year / 400))) % 7;
month = 5;
// 如果计算出的日期在当月之前,则将月份增加一个月
if (day <= 0) {
month++;
day += 7;
}
printf("%d年的母亲节日期为:%d月%d日", year, month, day);
return 0;
}
```
程序中使用了基姆拉尔森计算公式来计算母亲节的日期。公式中的参数year为输入的年份,计算出的日期存储在变量day和month中。如果计算出的日期在当月之前,则将月份增加一个月。最后输出计算结果,格式为“年份-月份-日期”。
母亲节是每年的五月第二个星期天,针对输入的年份,计算该年的哪一天是母亲节。帮我做一下这道题谢谢,用C语言写出代码
好的,我可以帮你编写一个简单的C语言函数来计算特定年份的母亲节日期。假设我们已经有一个包含`day_of_week`函数来获取给定日期是一周中的哪一天,这里是一个示例:
```c
#include <stdio.h>
#include <stdlib.h> // 使用这个头文件以获取time_t
// 获取给定日期是一周中的哪一天
int day_of_week(time_t date) {
return (date + 6) % 7; // 根据Unix时间戳规则,周一为第一天,返回值范围0-6
}
// 计算母亲节日期
void calculate_mother_day(int year) {
time_t t = mktime(&tm_year, 5, 2, 0, 0, 0, 0, -1, year); // 创建一个时间元组表示5月2日
int mother_day_date = day_of_week(t) + 1; // 从星期日开始计数,加上一天
int days_to_second_sunday;
// 如果5月2日已经是第二个周日,则不需要加额外天数
if (mother_day_date >= 7) {
days_to_second_sunday = (7 - mother_day_date) % 7;
} else {
// 计算到下一个完整的星期
days_to_second_sunday = (14 - mother_day_date) % 7;
}
printf("在年份 %d,母亲节是5月%d日。\n", year, 2 + days_to_second_sunday);
}
int main() {
int year;
printf("请输入年份: ");
scanf("%d", &year);
calculate_mother_day(year);
return 0;
}
```
当你运行这个程序并输入年份时,它将打印出对应年份的母亲节日期。
阅读全文
相关推荐
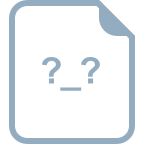

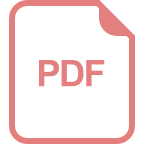
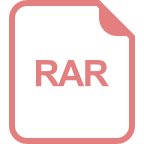
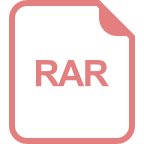
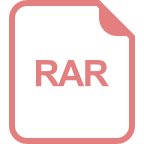
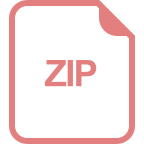
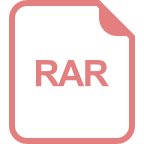
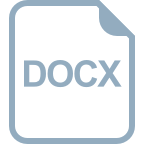
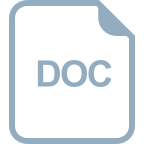
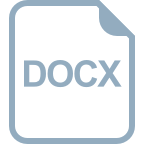
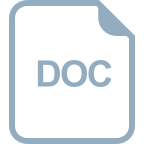