通过TensorFlow框架搭建GRU模型实现对IMDB数据集的分析的代码
时间: 2024-02-21 18:01:54 浏览: 87
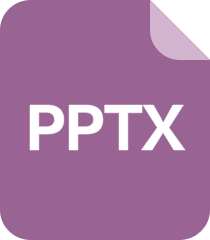
自动写诗ppt汇报.pptx
以下是使用TensorFlow框架搭建GRU模型实现对IMDB数据集的分析的完整代码:
```python
import tensorflow as tf
from tensorflow.keras.datasets import imdb
from tensorflow.keras.preprocessing.sequence import pad_sequences
# 加载IMDB数据集
vocab_size = 10000
max_len = 200
(X_train, y_train), (X_test, y_test) = imdb.load_data(num_words=vocab_size)
# 填充序列
X_train = pad_sequences(X_train, maxlen=max_len, padding='post', truncating='post')
X_test = pad_sequences(X_test, maxlen=max_len, padding='post', truncating='post')
# 构建GRU模型
model = tf.keras.Sequential([
tf.keras.layers.Embedding(vocab_size, 32),
tf.keras.layers.GRU(32, dropout=0.2, recurrent_dropout=0.5),
tf.keras.layers.Dense(1, activation='sigmoid')
])
# 编译模型
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
model.fit(X_train, y_train, epochs=10, batch_size=128, validation_data=(X_test, y_test))
# 在测试集上评估模型性能
score, acc = model.evaluate(X_test, y_test, batch_size=128)
print('Test score:', score)
print('Test accuracy:', acc)
```
在代码中,我们首先通过`imdb.load_data()`函数加载IMDB数据集,并将数据集中的单词数限制在10000个。然后,我们使用`pad_sequences()`函数将序列数据填充到相同的长度。接着,我们使用`tf.keras.Sequential()`函数构建模型,模型包含一个嵌入层、一个GRU层和一个全连接层。在构建模型后,我们使用`model.compile()`函数编译模型,指定损失函数、优化器和评估指标。最后,我们使用`model.fit()`函数训练模型,指定训练数据、训练轮数、批次大小和验证集。在训练完成后,我们使用`model.evaluate()`函数在测试集上评估模型的性能。
阅读全文
相关推荐
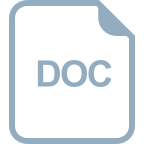
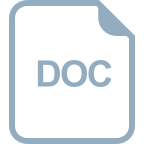





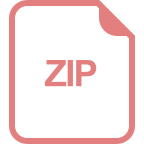
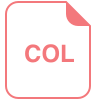
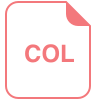
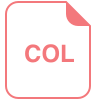
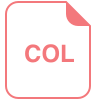
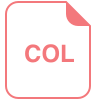
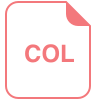
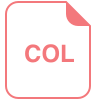
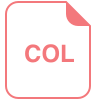
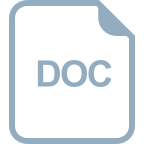
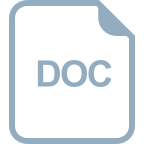