请帮我写出基于PYG搭建的GIN实现图分类的代码
时间: 2023-11-22 16:56:57 浏览: 157
好的,下面是基于PyG搭建的GIN实现图分类的代码。
首先,需要安装PyG和其他必要的库:
```python
!pip install torch
!pip install torch-geometric
!pip install torch-scatter
!pip install torch-sparse
!pip install torch-cluster
```
接下来,导入必要的库和数据集:
```python
import torch
import torch.nn.functional as F
from torch_geometric.datasets import Planetoid
from torch_geometric.nn import GINConv, global_mean_pool
# 加载数据集
dataset = 'Cora'
path = './data'
dataset = Planetoid(path, dataset)
```
然后,定义GIN模型:
```python
class GIN(torch.nn.Module):
def __init__(self, num_features, num_classes):
super(GIN, self).__init__()
# 定义GINConv层
self.conv1 = GINConv(torch.nn.Sequential(
torch.nn.Linear(num_features, 64),
torch.nn.BatchNorm1d(64),
torch.nn.ReLU(),
torch.nn.Linear(64, 64),
torch.nn.BatchNorm1d(64),
torch.nn.ReLU(),
torch.nn.Linear(64, 64),
torch.nn.BatchNorm1d(64),
torch.nn.ReLU(),
), train_eps=True)
self.conv2 = GINConv(torch.nn.Sequential(
torch.nn.Linear(64, 64),
torch.nn.BatchNorm1d(64),
torch.nn.ReLU(),
torch.nn.Linear(64, 64),
torch.nn.BatchNorm1d(64),
torch.nn.ReLU(),
torch.nn.Linear(64, 64),
torch.nn.BatchNorm1d(64),
torch.nn.ReLU(),
), train_eps=True)
# 定义全连接层
self.fc1 = torch.nn.Linear(64, 128)
self.fc2 = torch.nn.Linear(128, num_classes)
def forward(self, x, edge_index):
# 第一层GINConv
x = F.relu(self.conv1(x, edge_index))
x = F.relu(self.conv2(x, edge_index))
# 全局平均池化
x = global_mean_pool(x, edge_index)
x = F.relu(self.fc1(x))
x = F.dropout(x, p=0.5, training=self.training)
x = self.fc2(x)
return F.log_softmax(x, dim=1)
```
最后,定义训练函数和测试函数:
```python
def train(model, optimizer, data):
model.train()
optimizer.zero_grad()
out = model(data.x, data.edge_index)
loss = F.nll_loss(out[data.train_mask], data.y[data.train_mask])
loss.backward()
optimizer.step()
return loss.item()
def test(model, data):
model.eval()
out = model(data.x, data.edge_index)
pred = out.argmax(dim=1)
test_correct = pred[data.test_mask] == data.y[data.test_mask]
test_acc = int(test_correct.sum()) / int(data.test_mask.sum())
return test_acc
```
最后,训练和测试模型:
```python
# 定义模型、优化器和超参数
model = GIN(dataset.num_features, dataset.num_classes)
optimizer = torch.optim.Adam(model.parameters(), lr=0.01, weight_decay=5e-4)
epochs = 200
for epoch in range(1, epochs+1):
loss = train(model, optimizer, dataset[0])
acc = test(model, dataset[0])
print('Epoch {:03d}, Loss: {:.4f}, Test Acc: {:.4f}'.format(epoch, loss, acc))
```
这样,就完成了基于PyG搭建的GIN实现图分类的代码。
阅读全文
相关推荐
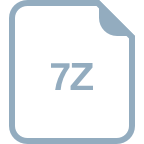


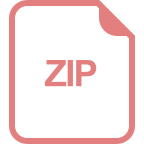
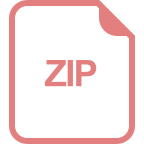






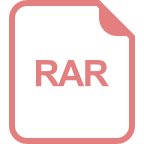
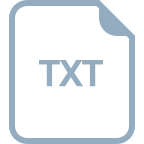
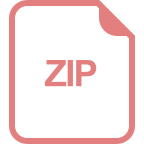