除了pmap还有哪些命令
时间: 2023-07-12 11:00:32 浏览: 101
除了 `pmap` 命令,Linux 系统中还有很多其他命令可以用来查看进程占用的内存情况,例如:
1. `ps` 命令:输入 `ps aux` 命令可以查看所有进程的详细信息,其中 `RSS` 表示进程实际占用的物理内存大小,`VSZ` 表示进程使用的虚拟内存大小。
2. `top` 命令:输入 `top` 命令后,可以按下 `M` 键,按照内存使用量排序,显示进程的内存占用情况。
3. `smem` 命令:输入 `smem -P <pid>` 命令,可以查看指定进程的内存占用情况,包括物理内存和虚拟内存的使用情况。
4. `pidstat` 命令:输入 `pidstat -r <interval> <count> -p <pid>` 命令,可以实时查看指定进程的内存占用情况。
这些命令都可以用来查看进程占用的内存情况,具体选择哪个命令取决于你的需求和习惯。
相关问题
c++代码实现linux命令pmap
以下是使用 C++ 实现 `pmap` 命令的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <sstream>
#include <iomanip>
using namespace std;
string to_hex(unsigned long long value) {
stringstream stream;
stream << "0x" << setfill('0') << setw(sizeof(void*)*2) << hex << value;
return stream.str();
}
int main(int argc, char** argv) {
if (argc != 2) {
cout << "Usage: " << argv[0] << " <process-id>" << endl;
return 1;
}
ifstream ifs("/proc/" + string(argv[1]) + "/maps");
if (!ifs) {
cout << "Failed to open /proc/" << argv[1] << "/maps" << endl;
return 2;
}
string line;
while (getline(ifs, line)) {
unsigned long long start, end;
string perms, offset, device, inode, pathname;
istringstream iss(line);
iss >> hex >> start;
iss.ignore(1, '-');
iss >> hex >> end;
iss >> perms >> offset >> device >> inode >> pathname;
cout << to_hex(start) << "-" << to_hex(end) << " " << perms << " " << offset << " " << device << " " << inode;
if (!pathname.empty()) {
cout << " " << pathname;
}
cout << endl;
}
return 0;
}
```
该程序会读取指定进程的 `/proc/<pid>/maps` 文件,解析其中的每一行内容,并将其输出到标准输出。输出的格式与 `pmap` 命令相同。
c语言实现命令pmap的功能
命令pmap可以查看一个进程的内存映射表,C语言可以通过读取/proc/[pid]/maps文件来实现类似的功能。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <pid>\n", argv[0]);
return 1;
}
char buf[MAX_LINE_LEN];
snprintf(buf, MAX_LINE_LEN, "/proc/%s/maps", argv[1]);
FILE *fp = fopen(buf, "r");
if (fp == NULL) {
printf("Failed to open %s\n", buf);
return 1;
}
while (fgets(buf, MAX_LINE_LEN, fp) != NULL) {
printf("%s", buf);
}
fclose(fp);
return 0;
}
```
这个程序接受一个进程ID作为参数,然后打开/proc/[pid]/maps文件,读取其中的内容并输出到标准输出。每一行的格式如下:
```
address perms offset dev inode pathname
```
其中,address表示内存地址范围,perms表示权限,offset表示偏移量,dev和inode表示设备号和inode编号,pathname表示映射的文件路径(可能为空)。
阅读全文
相关推荐
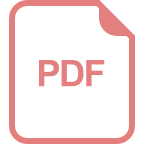
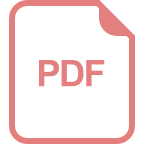
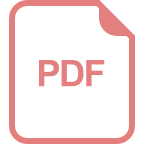












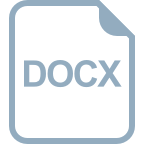
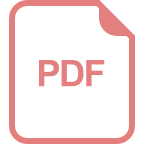