基于DenseNet的乳腺癌病理图像代码
时间: 2023-12-28 22:47:44 浏览: 50
以下是基于DenseNet的乳腺癌病理图像分类的代码示例:
```
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import os
import cv2
from sklearn.utils import shuffle
from sklearn.metrics import accuracy_score, confusion_matrix
from sklearn.model_selection import train_test_split
from keras.utils.np_utils import to_categorical
from keras.models import Sequential
from keras.layers import Dense, Dropout, Flatten, Conv2D, MaxPool2D, BatchNormalization
from keras.optimizers import RMSprop, Adam
from keras.preprocessing.image import ImageDataGenerator
from keras.callbacks import ReduceLROnPlateau, EarlyStopping
from keras.applications.densenet import DenseNet121
# 加载数据集
def load_data():
data_dir = '../input/breast-histopathology-images/IDC_regular_ps50_idx5/'
patients = os.listdir(data_dir)
labels = pd.read_csv('../input/breast-histopathology-images/IDC_regular_ps50_idx5/label.csv')
labels = pd.DataFrame({'path': labels['path'].apply(lambda x: x[4:]), 'label': labels['label']})
labels['id'] = labels['path'].apply(lambda x: x.split('/')[0])
labels['id'] = labels['id'].astype(int)
labels = labels.set_index('id')
labels = labels.loc[patients]
labels = labels.reset_index(drop=True)
labels = labels.drop(columns=['path'])
images = []
for patient in patients:
for file in os.listdir(data_dir + patient):
image = cv2.imread(data_dir + patient + '/' + file)
image = cv2.resize(image, (50, 50))
images.append(image)
images = np.array(images)
images, labels = shuffle(images, labels, random_state=42)
return images, labels
# 数据预处理
def preprocess_data(images, labels):
images = images / 255.0
labels = to_categorical(labels, num_classes=2)
x_train, x_test, y_train, y_test = train_test_split(images, labels, test_size=0.2, random_state=42)
return x_train, x_test, y_train, y_test
# 构建模型
def build_model():
densenet = DenseNet121(include_top=False, input_shape=(50, 50, 3))
model = Sequential()
model.add(densenet)
model.add(Flatten())
model.add(Dense(256, activation='relu'))
model.add(BatchNormalization())
model.add(Dropout(0.5))
model.add(Dense(2, activation='softmax'))
optimizer = Adam(lr=0.001)
model.compile(optimizer=optimizer, loss='categorical_crossentropy', metrics=['accuracy'])
return model
# 训练模型
def train_model(x_train, x_test, y_train, y_test):
datagen = ImageDataGenerator(rotation_range=10, zoom_range=0.1, width_shift_range=0.1, height_shift_range=0.1)
datagen.fit(x_train)
reduce_lr = ReduceLROnPlateau(monitor='val_accuracy', patience=3, min_lr=0.00001, verbose=1)
early_stop = EarlyStopping(monitor='val_loss', patience=5, verbose=1)
history = model.fit_generator(datagen.flow(x_train, y_train, batch_size=32), epochs=50, validation_data=(x_test, y_test),
verbose=1, steps_per_epoch=x_train.shape[0] // 32, callbacks=[reduce_lr, early_stop])
return history
# 可视化训练过程
def plot_history(history):
sns.set()
fig, ax = plt.subplots(1, 2, figsize=(20, 5))
ax[0].plot(history.history['loss'], label='Train Loss')
ax[0].plot(history.history['val_loss'], label='Validation Loss')
ax[0].set_xlabel('Epochs')
ax[0].set_ylabel('Loss')
ax[0].set_title('Loss')
ax[0].legend(loc='best')
ax[1].plot(history.history['accuracy'], label='Train Accuracy')
ax[1].plot(history.history['val_accuracy'], label='Validation Accuracy')
ax[1].set_xlabel('Epochs')
ax[1].set_ylabel('Accuracy')
ax[1].set_title('Accuracy')
ax[1].legend(loc='best')
plt.show()
# 测试模型
def test_model(x_test, y_test):
y_pred = model.predict(x_test)
y_pred = np.argmax(y_pred, axis=1)
y_test = np.argmax(y_test, axis=1)
accuracy = accuracy_score(y_test, y_pred)
cm = confusion_matrix(y_test, y_pred)
print('Test Accuracy:', accuracy)
print('Confusion Matrix:')
print(cm)
# 运行程序
images, labels = load_data()
x_train, x_test, y_train, y_test = preprocess_data(images, labels)
model = build_model()
history = train_model(x_train, x_test, y_train, y_test)
plot_history(history)
test_model(x_test, y_test)
```
以上代码使用DenseNet121作为卷积神经网络的基础模型,通过数据增强和迁移学习训练乳腺癌病理图像分类模型。
相关推荐
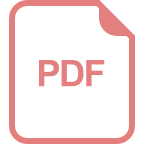
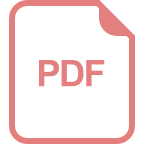
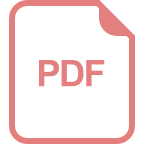














