springboot+mybitisplus如何从mysql中读取二进制文件并且返回给客户端,具体代码案列怎么写
时间: 2023-05-30 20:05:16 浏览: 559
以下是一个简单的 Spring Boot MyBatis Plus 示例,用于从 MySQL 中读取二进制文件并将其返回给客户端。该示例使用了 MyBatis Plus 的 `Blob` 类型和 `Base64` 编码方式。
在实体类中定义一个 `Blob` 类型的属性 `data`,用于存储二进制文件:
```java
@Data
public class FileEntity {
private Long id;
private String name;
private Blob data;
}
```
在 MyBatis Plus 的 Mapper 中添加一个查询方法,用于根据文件名获取文件数据:
```java
public interface FileMapper extends BaseMapper<FileEntity> {
@Select("SELECT * FROM file WHERE name = #{name}")
FileEntity selectByName(String name);
}
```
在控制器中编写一个方法,用于调用 Mapper 中的查询方法并将数据转换为 Base64 字符串后返回给客户端:
```java
@RestController
public class FileController {
@Autowired
private FileMapper fileMapper;
@GetMapping("/file/{name}")
public String getFileData(@PathVariable String name) throws IOException {
FileEntity file = fileMapper.selectByName(name);
if (file != null) {
byte[] bytes = file.getData().getBytes(1, (int) file.getData().length());
return Base64.getEncoder().encodeToString(bytes);
} else {
throw new FileNotFoundException("File not found: " + name);
}
}
}
```
在上述代码中,`getBytes` 方法用于将 `Blob` 类型的数据转换为字节数组,然后通过 Base64 编码方式将字节数组转换为字符串后返回给客户端。如果查询结果为空,则抛出 `FileNotFoundException` 异常。
阅读全文
相关推荐
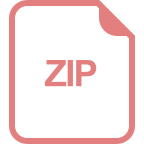
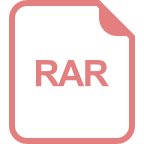
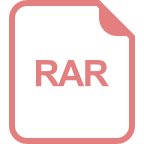
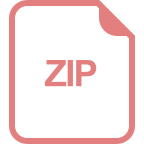
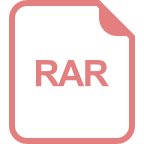
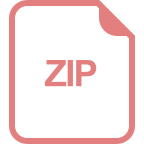
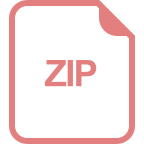
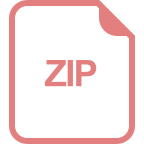
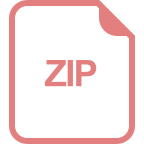
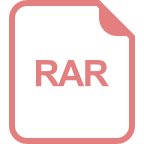
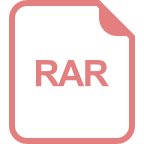
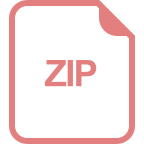
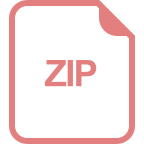
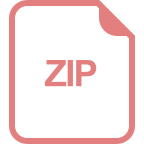
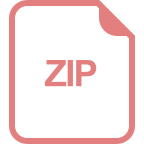
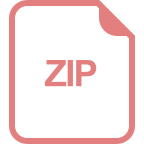
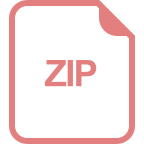