用javaweb编写一个超市付款系统
时间: 2023-08-22 16:08:23 浏览: 85
好的,下面给出一个简单的超市付款系统的实现,使用Java Web技术,包括JSP、Servlet和MySQL数据库。
1. 系统架构
超市付款系统主要由以下模块组成:
- 用户模块:包括注册、登录、修改密码等功能;
- 商品模块:包括商品列表、商品详情、商品分类等功能;
- 购物车模块:包括添加商品到购物车、修改购物车商品数量、删除购物车商品等功能;
- 订单模块:包括提交订单、我的订单、订单详情等功能;
- 支付模块:包括在线支付、货到付款等功能。
2. 技术选型
- 服务器:Tomcat
- 数据库:MySQL
- 后台框架:Spring MVC
- 前端框架:Bootstrap
- ORM框架:MyBatis
3. 数据库设计
超市付款系统需要设计的数据库表包括:
- 用户表:存储用户基本信息
- 商品表:存储商品基本信息
- 购物车表:存储用户购物车信息
- 订单表:存储用户订单信息
- 订单商品表:存储订单商品信息
具体的数据库设计可以参考以下SQL语句:
```
CREATE TABLE `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(50) NOT NULL COMMENT '用户名',
`password` varchar(50) NOT NULL COMMENT '密码',
`email` varchar(50) NOT NULL COMMENT '邮箱',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE `product` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL COMMENT '商品名称',
`price` decimal(10,2) NOT NULL COMMENT '商品价格',
`category_id` int(11) NOT NULL COMMENT '商品分类ID',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE `cart` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) NOT NULL COMMENT '用户ID',
`product_id` int(11) NOT NULL COMMENT '商品ID',
`quantity` int(11) NOT NULL COMMENT '数量',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE `order` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) NOT NULL COMMENT '用户ID',
`total_price` decimal(10,2) NOT NULL COMMENT '订单总金额',
`status` int(11) NOT NULL COMMENT '订单状态(0:待支付,1:已支付)',
`create_time` datetime NOT NULL COMMENT '订单创建时间',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE `order_product` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`order_id` int(11) NOT NULL COMMENT '订单ID',
`product_id` int(11) NOT NULL COMMENT '商品ID',
`price` decimal(10,2) NOT NULL COMMENT '单价',
`quantity` int(11) NOT NULL COMMENT '数量',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
4. 后端实现
在后端实现中,我们需要使用Spring MVC框架来处理HTTP请求,并使用MyBatis框架来访问数据库。
首先,我们需要创建一个UserController类来处理用户相关的HTTP请求:
```java
@Controller
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
/**
* 用户注册
*/
@RequestMapping(value = "/register", method = RequestMethod.POST)
public String register(User user, ModelMap model) {
if (userService.exist(user.getUsername())) {
model.put("error", "用户名已存在");
return "register";
}
userService.register(user);
return "redirect:/user/login";
}
/**
* 用户登录
*/
@RequestMapping(value = "/login", method = RequestMethod.POST)
public String login(User user, HttpSession session, ModelMap model) {
User loginUser = userService.login(user);
if (loginUser == null) {
model.put("error", "用户名或密码错误");
return "login";
}
session.setAttribute("user", loginUser);
return "redirect:/product/list";
}
/**
* 用户退出登录
*/
@RequestMapping(value = "/logout", method = RequestMethod.GET)
public String logout(HttpSession session) {
session.removeAttribute("user");
return "redirect:/product/list";
}
/**
* 修改密码
*/
@RequestMapping(value = "/changePassword", method = RequestMethod.POST)
public String changePassword(String oldPassword, String newPassword, HttpSession session, ModelMap model) {
User user = (User) session.getAttribute("user");
if (!oldPassword.equals(user.getPassword())) {
model.put("error", "旧密码错误");
return "changePassword";
}
user.setPassword(newPassword);
userService.update(user);
session.removeAttribute("user");
return "redirect:/user/login";
}
}
```
其中,我们使用了@Autowired注解来自动注入UserService实例,UserService类中封装了对User表的CRUD操作。
接下来,我们需要创建一个ProductController类来处理商品相关的HTTP请求:
```java
@Controller
@RequestMapping("/product")
public class ProductController {
@Autowired
private ProductService productService;
/**
* 商品列表
*/
@RequestMapping(value = "/list", method = RequestMethod.GET)
public String list(ModelMap model) {
List<Product> products = productService.getAll();
model.put("products", products);
return "productList";
}
/**
* 商品详情
*/
@RequestMapping(value = "/detail", method = RequestMethod.GET)
public String detail(int id, ModelMap model) {
Product product = productService.getById(id);
model.put("product", product);
return "productDetail";
}
}
```
在ProductController中,我们同样使用了@Autowired注解来自动注入ProductService实例,ProductService类中封装了对Product表的CRUD操作。
最后,我们需要创建一个CartController类来处理购物车相关的HTTP请求:
```java
@Controller
@RequestMapping("/cart")
public class CartController {
@Autowired
private CartService cartService;
/**
* 添加商品到购物车
*/
@RequestMapping(value = "/add", method = RequestMethod.POST)
@ResponseBody
public String add(int productId, int quantity, HttpSession session) {
User user = (User) session.getAttribute("user");
Cart cart = new Cart();
cart.setUserId(user.getId());
cart.setProductId(productId);
cart.setQuantity(quantity);
cartService.add(cart);
return "success";
}
/**
* 修改购物车商品数量
*/
@RequestMapping(value = "/update", method = RequestMethod.POST)
@ResponseBody
public String update(int id, int quantity) {
Cart cart = new Cart();
cart.setId(id);
cart.setQuantity(quantity);
cartService.update(cart);
return "success";
}
/**
* 删除购物车商品
*/
@RequestMapping(value = "/delete", method = RequestMethod.POST)
@ResponseBody
public String delete(int id) {
cartService.delete(id);
return "success";
}
/**
* 购物车列表
*/
@RequestMapping(value = "/list", method = RequestMethod.GET)
public String list(HttpSession session, ModelMap model) {
User user = (User) session.getAttribute("user");
List<CartVo> cartVos = cartService.getCartVoList(user.getId());
model.put("cartVos", cartVos);
return "cartList";
}
}
```
在CartController中,我们同样使用了@Autowired注解来自动注入CartService实例,CartService类中封装了对Cart表的CRUD操作。
5. 前端实现
在前端实现中,我们使用Bootstrap框架来构建页面,使用jQuery框架来处理交互事件。
首先,我们需要创建一个注册页面register.jsp:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>注册页面</title>
<link rel="stylesheet" href="${pageContext.request.contextPath}/static/css/bootstrap.min.css">
<script src="${pageContext.request.contextPath}/static/js/jquery.min.js"></script>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-4 col-md-offset-4">
<h3>注册</h3>
<form action="${pageContext.request.contextPath}/user/register" method="post">
<div class="form-group">
<label for="username">用户名:</label>
<input type="text" class="form-control" id="username" name="username" required>
</div>
<div class="form-group">
<label for="password">密码:</label>
<input type="password" class="form-control" id="password" name="password" required>
</div>
<div class="form-group">
<label for="email">邮箱:</label>
<input type="email" class="form-control" id="email" name="email" required>
</div>
<button type="submit" class="btn btn-primary">注册</button>
</form>
<p class="text-danger">${error}</p>
</div>
</div>
</div>
</body>
</html>
```
接下来,我们需要创建一个登录页面login.jsp:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>登录页面</title>
<link rel="stylesheet" href="${pageContext.request.contextPath}/static/css/bootstrap.min.css">
<script src="${pageContext.request.contextPath}/static/js/jquery.min.js"></script>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-4 col-md-offset-4">
<h3>登录</h3>
<form action="${pageContext.request.contextPath}/user/login" method="post">
<div class="form-group">
<label for="username">用户名:</label>
<input type="text" class="form-control" id="username" name="username" required>
</div>
<div class="form-group">
<label for="password">密码:</label>
<input type="password" class="form-control" id="password" name="password" required>
</div>
<button type="submit" class="btn btn-primary">登录</button>
</form>
<p class="text-danger">${error}</p>
</div>
</div>
</div>
</body>
</html>
```
然后,我们需要创建一个商品列表页面productList.jsp:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>商品列表</title>
<link rel="stylesheet" href="${pageContext.request.contextPath}/static/css/bootstrap.min.css">
<script src="${pageContext.request.contextPath}/static/js/jquery.min.js"></script>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-12">
<h3>商品列表</h3>
<table class="table table-bordered">
<thead>
<tr>
<th>ID</th>
<th>名称</th>
<th>价格</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${products}" var="product">
<tr>
<td>${product.id}</td>
<td>${product.name}</td>
<td>${product.price}</td>
<td><a href="${pageContext.request.contextPath}/product/detail?id=${product.id}">详情</a></td>
</tr>
</c:forEach>
</tbody>
</table>
</div>
</div>
</div>
</body>
</html>
```
最后,我们需要创建一个购物车页面cartList.jsp:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>购物车</title>
<link rel="stylesheet" href="${pageContext.request.contextPath}/static/css/bootstrap.min.css">
<script src="${pageContext.request.contextPath}/static/js/jquery.min.js"></script>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.min.js"></script>
<script>
$(function () {
$(".quantity").change(function () {
var id = $(this).attr("data-id");
var quantity = $(this).val();
$.post("${pageContext.request.contextPath}/cart/update", {id: id, quantity: quantity}, function () {
location.reload();
});
});
$(".delete").click(function () {
var id = $(this).attr("data-id");
$.post("${pageContext.request.contextPath}/cart/delete", {id: id}, function () {
location.reload();
});
});
});
</script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-12">
<h3>购物车</h3>
<table class="table table-bordered">
<thead>
<tr>
<th>ID</th>
<th>名称</th>
<th>价格</th>
<th>数量</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${cartVos}" var="cartVo">
<tr>
<td>${cartVo.product.id}</td>
<td>${cartVo.product.name}</td>
<td>${cartVo.product.price}</td>
<td><input type="number" class="form-control quantity" data-id="${cartVo.cart.id}" value="${cartVo.cart.quantity}" min="1"></td>
<td><button type="button" class="btn btn-danger delete" data-id="${cartVo.cart.id}">删除</button></td>
</tr>
</c:forEach>
</tbody>
</table>
<a href="${pageContext.request.contextPath}/order/create" class="btn btn-primary">提交订单</a>
</div>
</div>
</div>
</body>
</html>
```
6. 总结
超市付款系统是一个比较常见的电商网站,本文通过使用Java Web技术,实现了一个简单的超市付款系统,包括用户模块、商品模块、购物车模块、订单模块和支付模块等。
在后端实现中,我们使用了Spring MVC框架来处理HTTP请求,并使用MyBatis框架来访问数据库;在前端实现中,我们使用了Bootstrap框架来构建页面,使用jQuery框架来处理交互事件。
相关推荐
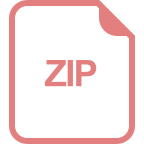
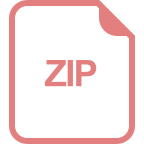
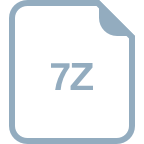
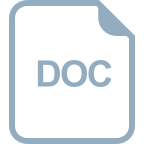
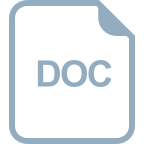
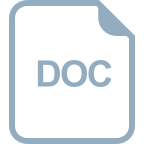
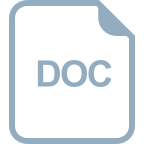
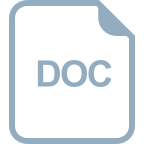
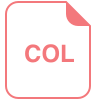
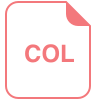
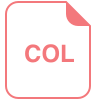
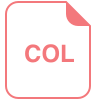
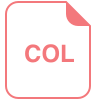




