编写一个程序,实现顺序表的初始化函数、插入数据元素函数、删除数据元素函数、查找函数,并编写主程序(main)调用函数实现顺序表的基本操作
时间: 2023-05-26 21:06:45 浏览: 92
#include<iostream>
using namespace std;
#define MaxSize 100
typedef struct{
int data[MaxSize];
int length;
}SqList;//顺序表类型定义
void InitList(SqList &L){
L.length=0;//初始化顺序表为空表
}//初始化函数
void CreateList(SqList &L,int n){
InitList(L);
for(int i=0;i<n;i++)
cin>>L.data[i];//输入n个元素
L.length=n;//表长为n
}//创建函数
int GetElem(SqList L,int i){
if(i<1||i>L.length) return -1;//判断是否越界
else return L.data[i-1];//返回第i个元素值
}//查找函数
int LocateElem(SqList L,int e){
for(int i=0;i<L.length;i++)
if(L.data[i]==e) return i+1;//返回e元素的位置序号
return 0;//查找失败
}//查找函数
bool ListInsert(SqList &L,int i,int e){
if(i<1||i>L.length+1) return false;//判断i的范围是否有效
if(L.length>=MaxSize) return false;//判断是否溢出
for(int j=L.length;j>=i;j--)
L.data[j]=L.data[j-1];//插入位置及之后的元素后移
L.data[i-1]=e;//插入e元素
L.length++;//表长加1
return true;
}//插入函数
bool ListDelete(SqList &L,int i,int &e){
if(i<1||i>L.length) return false;//判断i的范围是否有效
e=L.data[i-1];//e返回删除的元素
for(int j=i;j<L.length;j++)
L.data[j-1]=L.data[j];//删除位置之后的元素前移
L.length--;//表长减1
return true;
}//删除函数
void DispList(SqList L){
for(int i=0;i<L.length;i++)
cout<<L.data[i]<<" ";//输出所有元素值
cout<<endl;
}//输出函数
int main(){
SqList L;
int n,x,e,pos;
cout<<"请输入顺序表的长度:";
cin>>n;
cout<<"请依次输入所有元素:";
CreateList(L,n);
cout<<"插入元素操作,请输入插入位置和插入元素:";
cin>>pos>>x;
if(ListInsert(L,pos,x)) DispList(L);
else cout<<"插入失败!"<<endl;
cout<<"删除元素操作,请输入删除位置:";
cin>>pos;
if(ListDelete(L,pos,e)){
cout<<"删除的元素为:"<<e<<endl;
DispList(L);
}
else cout<<"删除失败!"<<endl;
cout<<"查找操作,请输入要查找的元素:";
cin>>x;
pos=LocateElem(L,x);
if(pos) cout<<"元素"<<x<<"的位置序号为:"<<pos<<endl;
else cout<<"查找失败!"<<endl;
return 0;
}
阅读全文
相关推荐
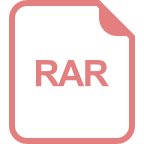


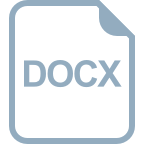
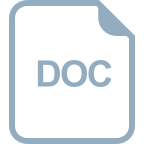
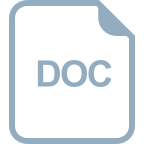
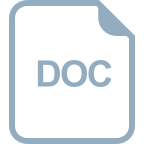
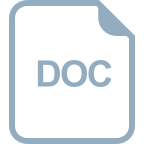
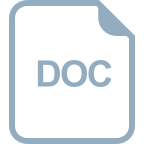
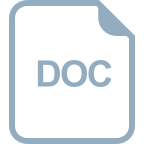
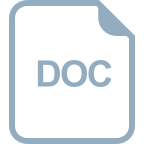
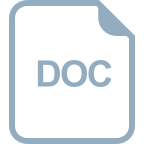
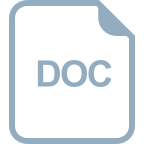
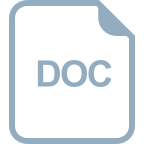
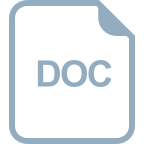
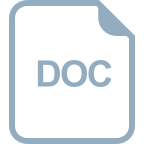