用C++模板写一个链表
时间: 2023-08-10 13:57:15 浏览: 135
当然可以!下面是一个使用C++模板编写的简单链表示例:
```cpp
#include <iostream>
template<typename T>
class Node {
public:
T data;
Node<T>* next;
Node(const T& value) : data(value), next(nullptr) {}
};
template<typename T>
class LinkedList {
private:
Node<T>* head;
public:
LinkedList() : head(nullptr) {}
void add(const T& value) {
Node<T>* newNode = new Node<T>(value);
if (head == nullptr) {
head = newNode;
} else {
Node<T>* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
void print() {
Node<T>* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
};
int main() {
LinkedList<int> myList;
myList.add(1);
myList.add(2);
myList.add(3);
myList.print();
return 0;
}
```
在这个示例中,我们定义了一个`Node`类来表示链表中的节点,其中包含数据(`data`)和指向下一个节点的指针(`next`)。然后,我们定义了一个`LinkedList`类来管理链表,它包括添加元素的`add`方法和打印链表的`print`方法。
在`main`函数中,我们创建一个整数类型的链表`myList`,并添加了三个元素。最后,我们调用`print`方法来打印整个链表。
请注意,这只是一个简单的示例,实际上链表可以有更多的操作和功能。这个示例可以作为你开始使用C++模板编写链表的起点,你可以根据需要进行修改和扩展。
阅读全文
相关推荐




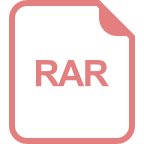








