1.创建表t_student,其中包含学号stuno,姓名stuname,性别stusex三个字段,在表中插入一些测试数据。(5分) 2.实现下述功能: 编写显示所有学生资料信息页面,其中包含一个表单用来根据学生姓名模糊查询匹配的
时间: 2024-02-13 13:06:55 浏览: 36
答案如下:
1. SQL语句如下:
```
CREATE TABLE t_student (
stuno INT PRIMARY KEY,
stuname VARCHAR(20),
stusex VARCHAR(2)
);
INSERT INTO t_student VALUES
(1, '张三', '男'),
(2, '李四', '女'),
(3, '王五', '男'),
(4, '赵六', '女'),
(5, '钱七', '男');
```
2. 实现功能的代码如下:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>学生信息管理系统</title>
</head>
<body>
<h1>学生信息管理系统</h1>
<form action="search.php" method="get">
<label for="name">请输入学生姓名:</label>
<input type="text" name="name" id="name" placeholder="请输入学生姓名">
<input type="submit" value="查询">
</form>
<br>
<table border="1">
<tr>
<th>学号</th>
<th>姓名</th>
<th>性别</th>
</tr>
<?php
// 连接数据库
$conn = mysqli_connect("localhost", "root", "password", "test");
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
// 查询学生信息
$sql = "SELECT * FROM t_student";
$result = mysqli_query($conn, $sql);
// 输出学生信息
if (mysqli_num_rows($result) > 0) {
while($row = mysqli_fetch_assoc($result)) {
echo "<tr>";
echo "<td>" . $row["stuno"] . "</td>";
echo "<td>" . $row["stuname"] . "</td>";
echo "<td>" . $row["stusex"] . "</td>";
echo "</tr>";
}
} else {
echo "<tr><td colspan='3'>暂无学生信息</td></tr>";
}
// 关闭数据库连接
mysqli_close($conn);
?>
</table>
</body>
</html>
```
```php
<?php
// 连接数据库
$conn = mysqli_connect("localhost", "root", "password", "test");
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
// 获取查询关键字
$name = $_GET["name"];
// 查询学生信息
$sql = "SELECT * FROM t_student WHERE stuname LIKE '%$name%'";
$result = mysqli_query($conn, $sql);
// 输出学生信息
if (mysqli_num_rows($result) > 0) {
echo "<table border='1'>";
echo "<tr><th>学号</th><th>姓名</th><th>性别</th></tr>";
while($row = mysqli_fetch_assoc($result)) {
echo "<tr>";
echo "<td>" . $row["stuno"] . "</td>";
echo "<td>" . $row["stuname"] . "</td>";
echo "<td>" . $row["stusex"] . "</td>";
echo "</tr>";
}
echo "</table>";
} else {
echo "暂无符合条件的学生信息";
}
// 关闭数据库连接
mysqli_close($conn);
?>
```
相关推荐
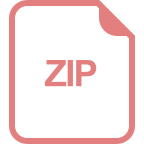
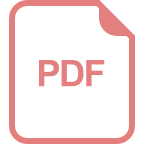
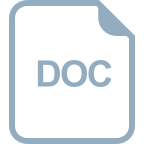














