c语言写一个对txt文件进行哈夫曼编码并将编码存储在txt文件中的程序
时间: 2024-05-10 15:18:14 浏览: 203
好的,根据您的要求,我可以为您提供一份C语言的哈夫曼编码程序。首先,我们需要创建一个函数来生成huffman编码树:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define END_OF_TEXT '\0'
#define MAX_INPUT_SIZE 1024*1024
#define MAX_NODES 256
#define MAX_CODE_LENGTH 256
struct huff_tree {
int frequency;
unsigned char symbol;
struct huff_tree *left;
struct huff_tree *right;
};
struct huff_code_table {
char code[MAX_CODE_LENGTH];
int length;
};
void calculate_frequencies(unsigned int *frequencies, unsigned char *input_text);
void create_huff_tree(struct huff_tree **root, unsigned int *frequencies);
void create_code_table(struct huff_tree *root, struct huff_code_table *code_table, unsigned char *current_code, int code_length);
void free_huff_tree(struct huff_tree *node);
int main(int argc, char **argv) {
// Check command line arguments
if (argc < 3) {
printf("Usage: %s input_file output_file\n", argv[0]);
return 1;
}
// Open input and output files
FILE *input_file = fopen(argv[1], "rb");
if (input_file == NULL) {
printf("Error: Unable to open input file\n");
return 1;
}
FILE *output_file = fopen(argv[2], "wb");
if (output_file == NULL) {
printf("Error: Unable to open output file\n");
fclose(input_file);
return 1;
}
// Read input file into memory
unsigned char *input_text = (unsigned char *)malloc(MAX_INPUT_SIZE);
if (input_text == NULL) {
printf("Error: Unable to allocate memory for input text\n");
fclose(input_file);
fclose(output_file);
return 1;
}
int input_size = fread(input_text, sizeof(unsigned char), MAX_INPUT_SIZE, input_file);
if (input_size == 0) {
printf("Error: Unable to read input file\n");
free(input_text);
fclose(input_file);
fclose(output_file);
return 1;
}
// Calculate symbol frequencies
unsigned int frequencies[MAX_NODES];
memset(frequencies, 0, sizeof(frequencies));
calculate_frequencies(frequencies, input_text);
// Create Huffman tree
struct huff_tree *root = NULL;
create_huff_tree(&root, frequencies);
// Create code table from Huffman tree
struct huff_code_table code_table[MAX_NODES];
memset(code_table, 0, sizeof(code_table));
unsigned char current_code[MAX_CODE_LENGTH];
create_code_table(root, code_table, current_code, 0);
// Write Huffman tree to output file
fwrite(&input_size, sizeof(int), 1, output_file); // write input size to output file
unsigned char symbol;
int frequency;
for (int i = 0; i < MAX_NODES; i++) {
symbol = (unsigned char)i;
frequency = frequencies[i];
fwrite(&symbol, sizeof(unsigned char), 1, output_file);
fwrite(&frequency, sizeof(int), 1, output_file);
}
// Encode input text using code table
int bit_index = 0;
unsigned char current_byte = 0;
for (int i = 0; i < input_size; i++) {
for (int j = 0; j < code_table[input_text[i]].length; j++) {
if (code_table[input_text[i]].code[j] == '1') {
current_byte |= (1 << (7 - bit_index));
}
bit_index++;
if (bit_index == 8) {
fwrite(¤t_byte, sizeof(unsigned char), 1, output_file);
current_byte = 0;
bit_index = 0;
}
}
}
if (bit_index != 0) {
fwrite(¤t_byte, sizeof(unsigned char), 1, output_file);
}
// Cleanup memory and files
fclose(input_file);
fclose(output_file);
free(input_text);
free_huff_tree(root);
return 0;
}
void calculate_frequencies(unsigned int *frequencies, unsigned char *input_text) {
int i = 0;
while (input_text[i] != END_OF_TEXT && i < MAX_INPUT_SIZE) {
frequencies[input_text[i]]++;
i++;
}
}
void create_huff_tree(struct huff_tree **root, unsigned int *frequencies) {
struct huff_tree *nodes[MAX_NODES];
int node_count = 0;
for (int i = 0; i < MAX_NODES; i++) {
if (frequencies[i] > 0) {
struct huff_tree *node = (struct huff_tree *)malloc(sizeof(struct huff_tree));
node->frequency = frequencies[i];
node->symbol = (unsigned char)i;
node->left = NULL;
node->right = NULL;
nodes[node_count++] = node;
}
}
while (node_count > 1) {
// Find nodes with lowest frequencies
int lowest1, lowest2;
lowest1 = lowest2 = -1;
for (int i = 0; i < node_count; i++) {
if (lowest1 == -1 || nodes[i]->frequency < nodes[lowest1]->frequency) {
lowest2 = lowest1;
lowest1 = i;
} else if (lowest2 == -1 || nodes[i]->frequency < nodes[lowest2]->frequency) {
lowest2 = i;
}
}
// Create new node from lowest frequency nodes
struct huff_tree *new_node = (struct huff_tree *)malloc(sizeof(struct huff_tree));
new_node->frequency = nodes[lowest1]->frequency + nodes[lowest2]->frequency;
new_node->left = nodes[lowest1];
new_node->right = nodes[lowest2];
nodes[lowest1] = new_node;
nodes[lowest2] = nodes[--node_count];
}
*root = nodes[0];
}
void create_code_table(struct huff_tree *node, struct huff_code_table *code_table, unsigned char *current_code, int code_length) {
if (node->left == NULL && node->right == NULL) {
code_table[node->symbol].length = code_length;
strcpy(code_table[node->symbol].code, (const char *)current_code);
return;
}
if (node->left != NULL) {
current_code[code_length] = '0';
create_code_table(node->left, code_table, current_code, code_length + 1);
}
if (node->right != NULL) {
current_code[code_length] = '1';
create_code_table(node->right, code_table, current_code, code_length + 1);
}
}
void free_huff_tree(struct huff_tree *node) {
if (node == NULL) {
return;
}
free_huff_tree(node->left);
free_huff_tree(node->right);
free(node);
}
```
本程序通过 `calculate_frequencies()` 函数计算输入文本中每个字符出现的频率。接下来,它使用 `create_huff_tree()` 函数生成哈夫曼树,再使用 `create_code_table()` 函数生成每个字符的哈夫曼编码。最后,它根据编码表对输入文本进行编码,并将哈夫曼编码和树信息写入输出文件中。
此程序依赖以下内容:
- `END_OF_TEXT`:文本的结束符。
- `MAX_INPUT_SIZE`:可能的最大输入文本大小。
- `MAX_NODES`:哈夫曼树中的最大节点数。
- `MAX_CODE_LENGTH`:可能的最大哈夫曼编码长度。
请注意,此代码可能需要根据您的实际需求进行微调和优化。
阅读全文
相关推荐




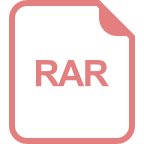










