poi实现excel转pdf 兼容xls,xlsx ,且自动转行
时间: 2024-01-06 13:04:27 浏览: 30
实现 Excel 转 PDF 兼容 xls 和 xlsx 格式的方法与上面的示例代码类似,需要使用 POI 读取 Excel 文件,然后根据不同的格式进行不同的处理。
对于自动转行,可以在写入 PDF 的时候,使用 PdfPTable 和 PdfPCell 对象来实现。具体的实现方法如下:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfPCell;
import com.itextpdf.text.pdf.PdfPTable;
import com.itextpdf.text.pdf.PdfWriter;
public class ExcelToPdf {
public static void main(String[] args) throws IOException, DocumentException {
String excelPath = "path/to/excel/file.xlsx";
String pdfPath = "path/to/pdf/file.pdf";
// 读取 Excel 文件
Workbook workbook = getWorkbook(excelPath);
// 创建 PDF 文件
Document document = new Document(PageSize.A4);
PdfWriter.getInstance(document, new FileOutputStream(pdfPath));
document.open();
// 遍历 Excel 中的每个 Sheet
for (int i = 0; i < workbook.getNumberOfSheets(); i++) {
Sheet sheet = workbook.getSheetAt(i);
int rowCount = sheet.getPhysicalNumberOfRows();
// 创建 PdfPTable 对象
PdfPTable table = new PdfPTable(sheet.getRow(0).getPhysicalNumberOfCells());
table.setWidthPercentage(100);
// 遍历 Sheet 中的每一行
for (int j = 0; j < rowCount; j++) {
Row row = sheet.getRow(j);
if (row != null) {
// 遍历每一列,将单元格内容添加到 PdfPCell 中
for (int k = 0; k < row.getPhysicalNumberOfCells(); k++) {
Cell cell = row.getCell(k);
if (cell != null) {
PdfPCell pdfPCell = new PdfPCell();
pdfPCell.setPhrase(new Paragraph(cell.toString()));
table.addCell(pdfPCell);
}
}
}
}
// 将 PdfPTable 添加到 PDF 中
document.add(table);
}
// 关闭 Workbook 和 Document 对象,保存 PDF 文件
workbook.close();
document.close();
}
private static Workbook getWorkbook(String excelPath) throws IOException {
Workbook workbook = null;
if (excelPath.endsWith(".xls")) {
workbook = new HSSFWorkbook(excelPath);
} else if (excelPath.endsWith(".xlsx")) {
workbook = new XSSFWorkbook(excelPath);
}
return workbook;
}
}
```
在上面的代码中,我们使用 getWorkbook 方法来获取 Excel 文件对应的 Workbook 对象。在遍历 Sheet 中的每一行和每一列时,我们将单元格内容添加到 PdfPCell 中,并将 PdfPCell 添加到 PdfPTable 中。由于 PdfPTable 对象会自动将内容转行,因此我们不需要手动进行转行的处理。最后,我们将 PdfPTable 对象添加到 PDF 文件中即可。
相关推荐
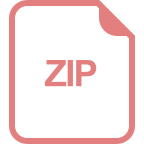
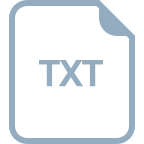














