用python写一段正则表达式,匹配规则如下,十八位纯数字的字符或者18位字符以x或X结尾
时间: 2024-05-03 19:23:17 浏览: 92
```
import re
pattern = r'^\d{18}|^\d{17}[xX]$'
string1 = '123456789012345678'
string2 = '12345678901234567x'
string3 = '12345678901234567X'
string4 = '1234567890123456789'
print(re.match(pattern, string1)) # <re.Match object; span=(0, 18), match='123456789012345678'>
print(re.match(pattern, string2)) # <re.Match object; span=(0, 18), match='12345678901234567x'>
print(re.match(pattern, string3)) # <re.Match object; span=(0, 18), match='12345678901234567X'>
print(re.match(pattern, string4)) # None
```
解释:
- `^` 匹配字符串的开头
- `\d` 匹配数字字符
- `{18}` 匹配前面的字符18次
- `|` 表示或者
- `\d{17}` 匹配前面的字符17次
- `[xX]` 匹配x或X
- `$` 匹配字符串的结尾
所以这个正则表达式可以匹配18位纯数字的字符或者18位字符以x或X结尾的字符串。
相关问题
python写一个正则表达式用于匹配整数
可以使用如下正则表达式来匹配整数:
```python
import re
num_regex = r'^-?\d+$'
# 匹配整数
if re.match(num_regex, '123'):
print('Matched')
else:
print('Not matched')
# 匹配负整数
if re.match(num_regex, '-456'):
print('Matched')
else:
print('Not matched')
# 匹配带符号整数
if re.match(num_regex, '+789'):
print('Matched')
else:
print('Not matched')
```
正则表达式 `^-?\d+$` 中,`^` 表示匹配字符串开头,`$` 表示匹配字符串结尾,`-?` 表示可选的负号,`\d+` 表示匹配一或多个数字。
Python使用正则表达式匹配字符串结尾
使用正则表达式匹配字符串结尾可以使用$符号。$符号匹配字符串的末尾。
示例代码:
```python
import re
string = "Hello World!"
pattern = r"World!$"
match = re.search(pattern, string)
if match:
print("字符串以 'World!' 结尾")
else:
print("字符串不以 'World!' 结尾")
```
输出:
```
字符串以 'World!' 结尾
```
在正则表达式中,$符号也可以用于匹配空行。例如,r"^$"匹配空行。
阅读全文
相关推荐
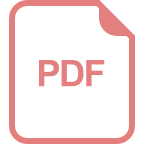


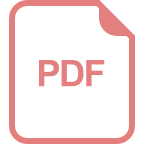
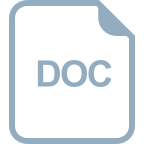
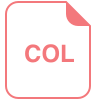
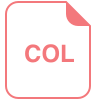
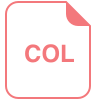
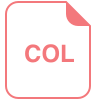
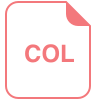




