基于 string filename = Path.GetFileName(image.FileName); string path = Server.MapPath("~/image/" + filename); 把path转成相对路径
时间: 2023-07-15 15:12:00 浏览: 157
可以使用相对路径的方式来获取目标路径,比如使用相对于当前应用程序根目录的路径,可以使用如下代码将绝对路径转换成相对路径:
```
string filename = Path.GetFileName(image.FileName);
string path = Server.MapPath("~/image/" + filename);
string relativePath = path.Replace(Server.MapPath("~/"), "~/").Replace("\\", "/");
```
这里使用 `Server.MapPath("~/")` 获取当前应用程序根目录的绝对路径,然后将目标路径中的绝对路径部分替换为相对路径部分,注意需要将反斜杠替换成正斜杠。最终得到的 `relativePath` 就是相对于当前应用程序根目录的路径。
相关问题
asp.net 将FileUpload1中的照片和其他信息一同存入sqlserver image字段中
在 ASP.NET 中,可以使用以下步骤将 FileUpload 控件中的图像和其他信息保存到 SQL Server 数据库中的 image 字段中:
1. 设置数据库连接字符串。
2. 在 ASP.NET 页面中添加 FileUpload 控件和其他输入控件(如文本框)来获取其他信息。
3. 在服务器端代码中,使用 FileUpload 控件的 SaveAs 方法将图像保存到服务器上的某个文件夹中。
4. 使用 System.IO.File 类的 ReadAllBytes 方法将保存的图像读入字节数组中。
5. 创建 SQL Server 数据库连接并打开连接。
6. 创建 SQL INSERT 语句,将图像字节数组和其他信息插入到数据库中的 image 字段中。
7. 执行 SQL INSERT 语句并关闭数据库连接。
以下是一个示例代码:
```csharp
protected void btnSubmit_Click(object sender, EventArgs e)
{
// 获取文件名和扩展名
string fileName = Path.GetFileName(FileUpload1.PostedFile.FileName);
string fileExtension = Path.GetExtension(FileUpload1.PostedFile.FileName);
// 保存文件到服务器上的某个文件夹中
string filePath = Server.MapPath("~/Images/") + fileName;
FileUpload1.SaveAs(filePath);
// 读入图像字节数组
byte[] imageData = File.ReadAllBytes(filePath);
// 获取其他信息
string name = txtName.Text;
string description = txtDescription.Text;
// 创建数据库连接
using (SqlConnection connection = new SqlConnection("your_connection_string_here"))
{
// 打开连接
connection.Open();
// 创建 SQL INSERT 语句
string sql = "INSERT INTO MyTable (Name, Description, ImageData) VALUES (@Name, @Description, @ImageData)";
// 创建 SqlCommand 对象
using (SqlCommand command = new SqlCommand(sql, connection))
{
// 添加参数
command.Parameters.AddWithValue("@Name", name);
command.Parameters.AddWithValue("@Description", description);
command.Parameters.AddWithValue("@ImageData", imageData);
// 执行 SQL INSERT 语句
command.ExecuteNonQuery();
}
// 关闭连接
connection.Close();
}
}
```
请注意,此示例代码仅供参考。您需要根据自己的实际情况对其进行修改。
asp.net中如何使用fileupload控件上传图片,上传后怎么显示在image控件上
可以通过以下步骤在 ASP.NET 中使用 FileUpload 控件上传图片并将其显示在 Image 控件上:
1. 在 ASP.NET 页面中添加 FileUpload 控件和 Image 控件。
2. 在页面的代码后台中添加以下代码:
```
protected void UploadButton_Click(object sender, EventArgs e)
{
if(FileUpload1.HasFile)
{
try
{
string filename = Path.GetFileName(FileUpload1.FileName);
FileUpload1.SaveAs(Server.MapPath("~/") + filename);
Image1.ImageUrl = "~/"+filename;
StatusLabel.Text = "Upload status: File uploaded!";
}
catch(Exception ex)
{
StatusLabel.Text = "Upload status: The file could not be uploaded. The following error occured: " + ex.Message;
}
}
}
```
3. 在代码后台中添加以下代码以处理 Image 控件的默认图像:
```
protected void Page_Load(object sender, EventArgs e)
{
if(!IsPostBack)
{
Image1.ImageUrl = "~/Images/default.jpg";
}
}
```
通过这些步骤,您可以在 ASP.NET 中使用 FileUpload 控件上传图片并将其显示在 Image 控件上。
阅读全文
相关推荐
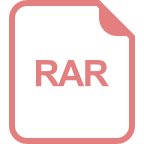
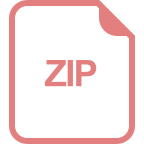
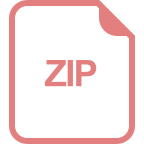
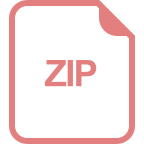
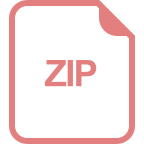
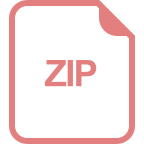
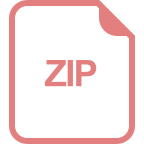
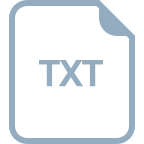
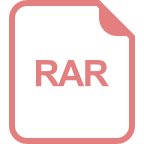
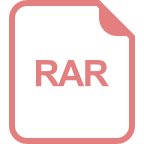
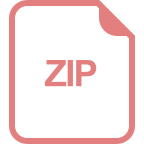
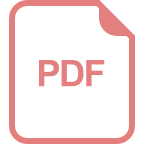
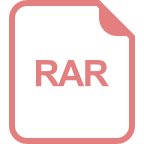
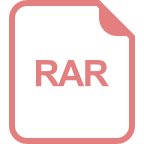