d3js饼状图
时间: 2023-08-06 12:12:33 浏览: 84
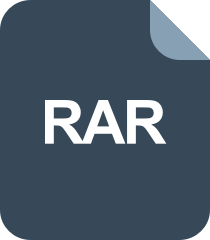
3D 饼状图
使用d3.js制作饼状图可以分为以下步骤:
1. 准备数据:需要准备一个包含每个部分所占比例的数据集。
2. 创建 SVG 元素:使用 d3.select() 和 append() 方法创建 SVG 元素。
3. 设置饼图参数:使用 d3.pie() 方法设置饼图参数,例如排序方式、起始角度、结束角度等。
4. 绘制饼图:使用 d3.arc() 方法绘制每个部分的路径,并使用 d3.select() 和 append() 方法创建 path 元素。
5. 添加标签:使用 d3.text() 方法添加标签,例如图例、标题等。
6. 添加交互:使用 d3.mouseover() 和 d3.mouseout() 方法为饼图添加鼠标事件。
以下是一个基本的饼状图代码示例:
```javascript
// 准备数据
var dataset = [30, 10, 20, 40];
// 创建 SVG 元素
var svg = d3.select("body")
.append("svg")
.attr("width", 400)
.attr("height", 400);
// 设置饼图参数
var pie = d3.pie()
.sort(null)
.value(function(d) { return d; });
// 绘制饼图
var arc = d3.arc()
.innerRadius(0)
.outerRadius(150);
var arcs = svg.selectAll("g.arc")
.data(pie(dataset))
.enter()
.append("g")
.attr("class", "arc")
.attr("transform", "translate(200,200)");
arcs.append("path")
.attr("d", arc)
.attr("fill", function(d, i) {
return d3.schemeCategory10[i];
});
// 添加标签
svg.append("text")
.attr("x", 180)
.attr("y", 20)
.attr("text-anchor", "middle")
.attr("font-size", "20px")
.text("饼状图");
// 添加交互
arcs.on("mouseover", function(d) {
d3.select(this)
.select("path")
.attr("stroke", "#fff")
.attr("stroke-width", 2);
})
.on("mouseout", function(d) {
d3.select(this)
.select("path")
.attr("stroke", "none");
});
```
在这个示例中,我们准备了一个包含四个部分的数据集,创建了一个 SVG 元素,设置了饼图参数,绘制了每个部分的路径,并添加了标签和交互。你可以根据自己的需求对代码进行修改和扩展。
阅读全文
相关推荐
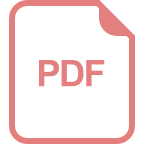
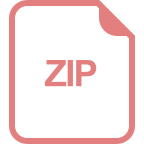
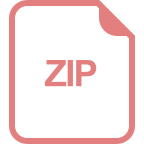
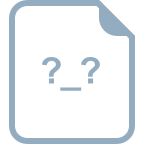
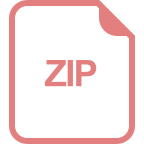
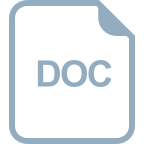
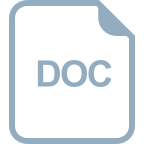
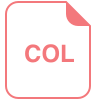
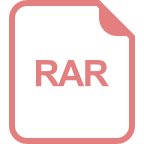
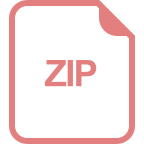
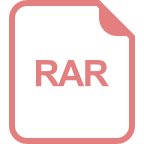
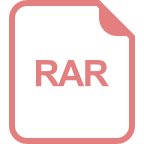
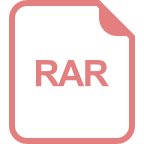
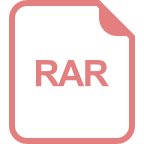
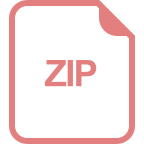
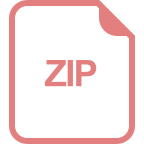
