python里面实现输出的方法
时间: 2023-05-04 14:05:08 浏览: 159
Python 有多种输出方法,下面介绍其中比较常用的几种:
1. 使用 print() 函数:是最基本的输出方法,可以将函数参数依次输出到控制台。例如:
```
print('Hello World!')
```
2. 使用 format() 方法:通过 {} 占位符和 format() 方法将变量插入到字符串中输出。例如:
```
name = 'Tom'
age = 18
print('{} is {} years old.'.format(name, age))
```
3. 使用 % 格式化字符串:类似于 C 语言的 printf() 函数,可以将变量插入到格式化字符串中输出。例如:
```
name = 'Tom'
age = 18
print('%s is %d years old.' % (name, age))
```
4. 使用 f-string:Python 3.6 版本新增的字符串格式化方法,使用 f 前缀,在字符串中通过 {} 插入变量。例如:
```
name = 'Tom'
age = 18
print(f'{name} is {age} years old.')
```
以上是 Python 中常见的输出方法,根据具体情况选择合适的方法进行输出。
相关问题
python输出里面选择输出的结果并输出
可以使用 Python 的输入输出函数 `input()` 和 `print()` 来实现选择输出的结果并输出。具体的实现方式如下:
1. 首先,你需要将所有可能的输出结果存储在一个列表或字典中,如下所示:
```
results = {
1: "Hello, World!",
2: "How are you?",
3: "Nice to meet you!"
}
```
2. 然后,使用 `input()` 函数来获取用户选择的结果,如下所示:
```
choice = int(input("请选择要输出的结果(1/2/3):"))
```
这里使用 `int()` 函数将用户输入的字符串转换为整数类型。
3. 最后,使用 `print()` 函数来输出用户选择的结果,如下所示:
```
print(results.get(choice, "无效的选择"))
```
这里使用 `get()` 方法来获取用户选择的结果,如果选择无效,则输出 "无效的选择"。
完整的代码如下所示:
```
results = {
1: "Hello, World!",
2: "How are you?",
3: "Nice to meet you!"
}
choice = int(input("请选择要输出的结果(1/2/3):"))
print(results.get(choice, "无效的选择"))
```
python里面prettytable按行输出数组
你可以使用 Python 的第三方库 PrettyTable 来实现按行输出数组,以下是一个示例代码:
```python
from prettytable import PrettyTable
# 创建一个 3x3 的二维数组
arr = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# 创建一个 PrettyTable 对象
table = PrettyTable()
# 添加表头
table.field_names = ["A", "B", "C"]
# 按行添加数据
for row in arr:
table.add_row(row)
# 输出表格
print(table)
```
输出结果如下:
```
+---+---+---+
| A | B | C |
+---+---+---+
| 1 | 2 | 3 |
| 4 | 5 | 6 |
| 7 | 8 | 9 |
+---+---+---+
```
你可以根据需要修改表头名称,以及调整表格的格式和样式。
阅读全文
相关推荐
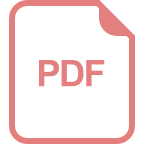
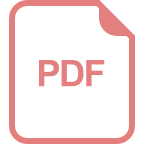
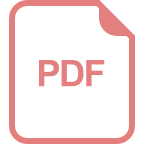
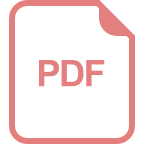
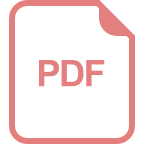
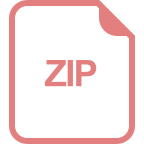
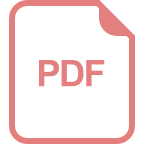
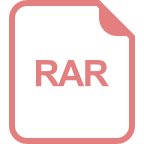
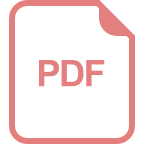
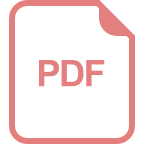





