设计自定义异常类NotTriangleException;设计Triangle(三角形)类; (1)Triangle类基本信息:三个顶点坐标; (2)带三个顶点坐标参数的构造函数;当传入实参为不合理数据时(例如:输入的三角形的顶点不能组成一个三角形),抛出自定义异常类NotTriangleException的对象; (3)有返回三角形面积的函数getArea()。 (4)各个类的声明放在与类同名的头文件中(*.h),类的实现放在与类同名的源文件中(*.cpp); (5)只能在主函数中输入输出,各个类的成员函数中不能进行输入输出。 (6)在主函数中,接收用户输入的三角形三个顶点坐标,然后,传给三角形对象的构造函数,并捕获NotTriangleException异常对象。当捕获到异常后,提示用户输入数据错误,并要求用户重新输入。
时间: 2023-12-03 17:45:03 浏览: 51
NotTriangleException.h
```c++
#ifndef NOTTRIANGLEEXCEPTION_H
#define NOTTRIANGLEEXCEPTION_H
#include <exception>
#include <string>
class NotTriangleException : public std::exception {
public:
NotTriangleException(const std::string& msg): message(msg) {}
virtual const char* what() const noexcept {
return message.c_str();
}
private:
std::string message;
};
#endif // NOTTRIANGLEEXCEPTION_H
```
Triangle.h
```c++
#ifndef TRIANGLE_H
#define TRIANGLE_H
#include "NotTriangleException.h"
class Triangle {
public:
Triangle(double x1, double y1, double x2, double y2, double x3, double y3);
double getArea() const;
private:
double x1, y1, x2, y2, x3, y3;
};
#endif // TRIANGLE_H
```
Triangle.cpp
```c++
#include "Triangle.h"
#include <cmath>
Triangle::Triangle(double x1, double y1, double x2, double y2, double x3, double y3) {
double a = sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2));
double b = sqrt(pow(x1 - x3, 2) + pow(y1 - y3, 2));
double c = sqrt(pow(x2 - x3, 2) + pow(y2 - y3, 2));
if (a + b <= c || a + c <= b || b + c <= a) {
throw NotTriangleException("Invalid triangle coordinates");
}
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
this->x3 = x3;
this->y3 = y3;
}
double Triangle::getArea() const {
double a = sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2));
double b = sqrt(pow(x1 - x3, 2) + pow(y1 - y3, 2));
double c = sqrt(pow(x2 - x3, 2) + pow(y2 - y3, 2));
double s = (a + b + c) / 2.0;
double area = sqrt(s * (s - a) * (s - b) * (s - c));
return area;
}
```
main.cpp
```c++
#include <iostream>
#include "Triangle.h"
using namespace std;
int main() {
double x1, y1, x2, y2, x3, y3;
while (true) {
cout << "Enter the coordinates of the triangle's vertices:" << endl;
cin >> x1 >> y1 >> x2 >> y2 >> x3 >> y3;
try {
Triangle t(x1, y1, x2, y2, x3, y3);
double area = t.getArea();
cout << "The area of the triangle is " << area << endl;
break;
} catch (NotTriangleException& e) {
cout << "Error: " << e.what() << endl;
cout << "Please enter valid coordinates." << endl;
}
}
return 0;
}
```
阅读全文
相关推荐
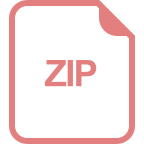


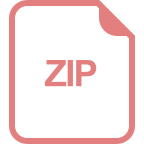
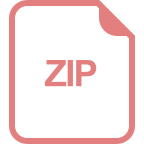
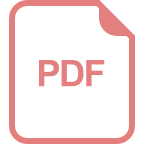
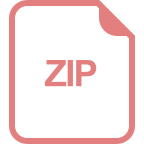
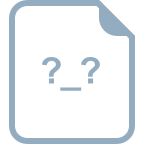
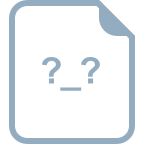
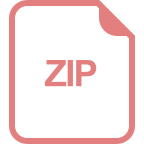
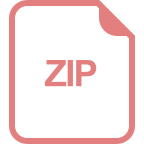
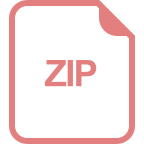



