当鼠标划走时,坐标显示应该消失
时间: 2024-03-23 15:38:00 浏览: 44
要实现鼠标离开网格时,坐标显示消失的功能,只需要在处理鼠标移动事件时,添加一个清除坐标显示的操作即可。具体步骤如下:
1. 在处理鼠标移动事件时,检查鼠标是否在网格内。
2. 如果鼠标在网格内,则显示坐标位置,并将其保存到一个变量中。
3. 如果鼠标已经离开了网格,则清除屏幕上之前显示的坐标位置。
以下是修改后的示例代码:
```python
import pygame
# 定义网格的边长、行数和列数
grid_size = 50
num_rows = 10
num_cols = 10
# 初始化Pygame
pygame.init()
# 创建屏幕对象
screen_width = num_cols * grid_size
screen_height = num_rows * grid_size
screen = pygame.display.set_mode((screen_width, screen_height))
# 绘制坐标轴
pygame.draw.line(screen, (255, 255, 255), (0, 0), (0, screen_height), 2) # 纵向坐标轴
pygame.draw.line(screen, (255, 255, 255), (0, 0), (screen_width, 0), 2) # 横向坐标轴
# 标注坐标
font = pygame.font.Font(None, 20)
for i in range(num_rows):
text = font.render(str(i), True, (255, 255, 255))
screen.blit(text, (5, i * grid_size + 5))
for j in range(num_cols):
text = font.render(str(j), True, (255, 255, 255))
screen.blit(text, (j * grid_size + 5, 5))
# 绘制网格
for i in range(num_rows):
for j in range(num_cols):
pygame.draw.rect(screen, (255, 255, 255), (j * grid_size, i * grid_size, grid_size, grid_size), 1)
# 刷新屏幕
pygame.display.flip()
# 进入消息循环
last_pos = None # 记录上一个显示的位置
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEMOTION:
# 获取鼠标当前的位置,并将其转换为网格的行列坐标
pos = pygame.mouse.get_pos()
col = pos[0] // grid_size
row = pos[1] // grid_size
# 检查鼠标是否在网格内
if 0 <= col < num_cols and 0 <= row < num_rows:
# 在屏幕上显示坐标位置,并记录该位置
text = font.render('({}, {})'.format(row, col), True, (255, 255, 255))
screen.blit(text, (col * grid_size + 5, row * grid_size + 5))
last_pos = (row, col)
else:
# 清除屏幕上之前显示的坐标位置
if last_pos is not None:
screen.fill((0, 0, 0), (last_pos[1] * grid_size, last_pos[0] * grid_size, grid_size, grid_size))
last_pos = None
# 刷新屏幕
pygame.display.flip()
```
上述代码会在鼠标滑动到网格时,在该网格的左上角显示坐标位置,并在鼠标离开网格时,清除之前显示的坐标位置。
阅读全文
相关推荐
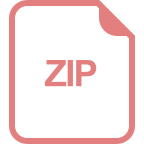
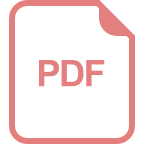
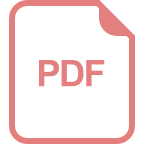















