Matplotlib 3D点云显示。鼠标移动到点上时显示其坐标
时间: 2024-03-12 21:48:46 浏览: 18
要实现在鼠标移动到点上时显示其坐标,可以利用 Matplotlib 的事件处理机制,监听鼠标移动事件,然后根据鼠标所在的位置,计算出其对应的点的坐标,并将其显示在图形上。
下面是一个简单的例子,演示了如何实现这个功能:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 生成随机数据
n = 50
x = np.random.rand(n)
y = np.random.rand(n)
z = np.random.rand(n)
# 创建 3D 图形对象
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 绘制 3D 散点图
scatter = ax.scatter(x, y, z, s=50, c=z, cmap='cool')
# 设置坐标轴标签
ax.set_xlabel('X Label')
ax.set_ylabel('Y Label')
ax.set_zlabel('Z Label')
# 添加交互功能
def on_move(event):
if event.inaxes == ax:
x, y = event.xdata, event.ydata
ind = np.argmin(np.sqrt((x - x)**2 + (y - y)**2))
scatter.set_sizes([50]*(n-1) + [300])
scatter.set_edgecolors(np.repeat('black', n))
scatter.set_facecolors(np.repeat('gray', n))
scatter.set_edgecolors(ind, 'red')
scatter.set_facecolors(ind, 'orange')
ax.text2D(0, 0, f'({x:.2f}, {y:.2f}, {z[ind]:.2f})', transform=ax.transAxes)
fig.canvas.mpl_connect('motion_notify_event', on_move)
plt.show()
```
这个例子中,我们使用了 Matplotlib 的 `motion_notify_event` 事件来监听鼠标移动事件。当鼠标移动到点上时,我们利用鼠标所在的位置计算出其对应的点的坐标,并将其显示在图形上。同时,我们还对该点进行了突出显示,以便用户更好地识别当前所在的点。
当你运行这段代码时,应该可以看到一个带有鼠标移动交互功能的 3D 散点图。当你将鼠标移动到点上时,该点的坐标会显示在图形上。
相关推荐
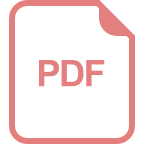














