opencv,自选图片,使用区域生长算法进行图像分割,请给出代码
时间: 2023-06-03 20:02:18 浏览: 115
以下是使用区域生长算法进行图像分割的 opencv 代码示例:
```python
import cv2
# 读取图片
img = cv2.imread('your_image.jpg')
# 区域生长算法
def region_growing(seed, input_img, threshold):
# 输入图像大小
height, width = input_img.shape[:2]
# 创建一个与输入图像相同大小的标记矩阵
segmented = np.zeros_like(input_img)
# 将种子点设置为已标记
segmented[seed] = 255
# 初始化队列
queue = []
queue.append(seed)
# 迭代进行区域生长
while queue:
# 取出队首像素点
current_index = queue.pop(0)
# 得到当前像素点的值
pixel_value = input_img[current_index[0], current_index[1]]
# 循环处理当前像素点的 8 个相邻点
for i in range(-1, 2):
for j in range(-1, 2):
# 跳过当前像素点自身和越界的点
if i == 0 and j == 0:
continue
x = current_index[0] + i
y = current_index[1] + j
if x < 0 or y < 0 or x >= height or y >= width:
continue
# 获取相邻点的像素值
neighbour_pixel_value = input_img[x, y]
# 如果相邻点没有被标记过并且与当前像素点相似
if segmented[x, y] == 0 and abs(int(neighbour_pixel_value) - int(pixel_value)) < threshold:
# 标记相邻点
segmented[x, y] = 255
# 将相邻点插入队列中,继续生长
queue.append([x, y])
return segmented
# 设置种子点
seed_x, seed_y = 50, 50
seed = [seed_x, seed_y]
# 执行区域生长算法
threshold = 10 # 阈值,用于控制生长的区域大小
seg = region_growing(seed, img, threshold)
# 显示原始图片和分割结果
cv2.imshow('Original', img)
cv2.imshow('Segmentation', seg)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意:以上代码仅为示例,实际使用时需要根据具体问题进行调整。
相关推荐
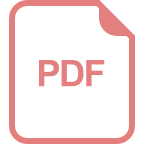
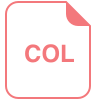
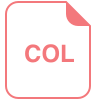
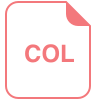
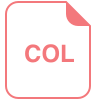
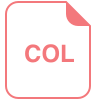









