#include<iostream> #include<cstring> using namespace std; class MyclassA{ public: MyclassA(char *s) { strcpy(str,s); } void print() { cout<<str<<endl; } protected: char str[100]; }; class MyclassB{ public: MyclassB(char *s) { strcpy(str,s); } void print() { cout<<str<<endl; } protected: char str[100]; }; class MyclassC:public MyclassA,public MyclassB{ public: MyclassC(char *s1,char *s2):MyclassA(s1),MyclassB(s2) { } void splicing() { strcpy(str,MyclassA::str); //ERROR**********found********** strcat(str,MyclassB::str); } void print() { cout<<str<<endl; } private: char str[200]; }; int main(void) { MyclassC test("stringA","stringB"); //ERROR**********found********** test.print(S); // 输出stringA //ERROR**********found********** test.print(s); // 输出stringB test.splicing(); test.print(); // 输出stringAstringB system("pause"); return 0; }错在哪
时间: 2023-07-07 14:44:37 浏览: 103
这段代码中有多处错误,我来一一指出并解释:
1. 在 MyclassC 的构造函数中,应该使用初始化列表来初始化继承的两个基类 MyclassA 和 MyclassB 中的数据成员,而不是在构造函数体内直接调用它们的构造函数来初始化。正确的构造函数应该是这样的:
```
MyclassC(char *s1, char *s2) : MyclassA(s1), MyclassB(s2) {}
```
2. 在 MyclassC 的 splicing 函数中,应该使用 MyclassA 和 MyclassB 中的 print 函数来分别输出它们各自的字符串,而不是直接访问它们的数据成员。正确的 splicing 函数应该是这样的:
```
void splicing() {
MyclassA::print();
MyclassB::print();
strcpy(str, MyclassA::str);
strcat(str, MyclassB::str);
}
```
3. 在 main 函数中,调用 MyclassC 的 print 函数时应该传入一个字符串字面量,而不是一个未定义的变量名。正确的调用应该是这样的:
```
test.print("S");
test.print("s");
```
4. 在 main 函数末尾,应该使用 `system("pause")` 来暂停程序的执行,否则程序会在输出结果后立即退出。
相关问题
#include <iostream> #include<algorithm> #include<cmath> #include <queue> using namespace std;
#include <iostream>:这是C++标准库中的头文件,用于输入输出流操作,包括cin、cout等。
#include<algorithm>:这是C++标准库中的头文件,用于提供各种算法操作,如排序、查找等。
#include<cmath>:这是C++标准库中的头文件,用于提供数学函数操作,如绝对值、平方根等。
#include <queue>:这是C++标准库中的头文件,用于提供队列操作,包括入队、出队等。
using namespace std;:这是C++的命名空间声明,表示使用std命名空间,可以直接使用std中的函数和对象。
#include <iostream> #include <string> #include <stack> #include <vector> #include <iomanip> using namespace std; 逐行注释以上代码
这是一段C++的程序代码,使用了几个标准库的头文件。以下是逐行的注释:
```
#include <iostream> // 输入输出流库头文件
#include <string> // 字符串库头文件
#include <stack> // 栈库头文件
#include <vector> // 向量库头文件
#include <iomanip> // 格式控制库头文件
using namespace std; // 命名空间
```
这段程序代码导入了五个标准库的头文件,分别是iostream、string、stack、vector、iomanip,它们分别用于处理输入输出、字符串、栈、向量和格式控制。其中iostream是C++中最常用的输入输出流库,string是用于字符串操作的库,stack是用于栈数据结构的库,vector是用于动态数组的库,iomanip是用于格式化输出的库。
最后一行代码使用了命名空间std,这意味着所有的标准库函数和变量都在std命名空间中。如果不使用using namespace std,则需要在使用标准库函数时加上std::前缀。
相关推荐
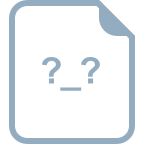













