Unity怎么使预制体上的代码控制预制体Ui的显示和关闭
时间: 2024-05-12 14:17:53 浏览: 14
Unity中可以通过在预制体上添加脚本来控制预制体UI的显示和关闭。在脚本中获取UI组件的引用,并在需要时设置其active属性为true或false即可。例如,通过以下代码实现预制体上Button的点击事件控制一个Text组件的显示和隐藏:
```csharp
public class PrefabController : MonoBehaviour
{
public GameObject textPrefab;
void Start()
{
// 在预制体上查找Button组件,并注册点击事件
Button button = GetComponent<Button>();
button.onClick.AddListener(() => {
// 在预制体上查找Text组件,并设置其显示状态
GameObject textObj = Instantiate(textPrefab);
textObj.transform.SetParent(transform.parent);
textObj.GetComponent<Text>().text = "Hello World!";
textObj.SetActive(true);
});
}
}
```
相关问题
unity如何自动生成ugui预制体 代码
在 Unity 中,可以使用以下 C# 代码自动生成 UGUI 预制体:
```csharp
using UnityEditor;
using UnityEngine;
using UnityEngine.UI;
public static class PrefabGenerator
{
[MenuItem("GameObject/Create UGUI Prefab")]
public static void CreateUIPrefab()
{
// 获取当前选择的 GameObject
GameObject selectedObject = Selection.activeGameObject;
// 创建一个新的 GameObject 作为存储 Prefab 的容器
GameObject prefabContainer = new GameObject(selectedObject.name + "Prefab");
// 将当前选择的 GameObject 的所有子物体复制到新的容器中
foreach (Transform child in selectedObject.transform)
{
GameObject childObject = Object.Instantiate(child.gameObject);
childObject.transform.SetParent(prefabContainer.transform);
}
// 添加 Canvas 组件和 Graphic Raycaster 组件
Canvas canvas = prefabContainer.AddComponent<Canvas>();
prefabContainer.AddComponent<GraphicRaycaster>();
// 设置 Canvas 属性
canvas.renderMode = RenderMode.ScreenSpaceOverlay;
canvas.sortingOrder = 0;
// 保存 Prefab
string path = "Assets/" + selectedObject.name + ".prefab";
Object prefab = PrefabUtility.SaveAsPrefabAsset(prefabContainer, path);
// 销毁容器 GameObject 和子物体
Object.DestroyImmediate(prefabContainer);
// 在 Project 视图中选中新创建的 Prefab
Selection.activeObject = prefab;
}
}
```
这段代码的作用是创建一个菜单项,可以通过在 Unity 编辑器中点击菜单栏的 GameObject -> Create UGUI Prefab 来自动生成 UGUI 预制体。在创建 Prefab 时,会将当前选择的 GameObject 的所有子物体复制到新的容器中,添加 Canvas 和 Graphic Raycaster 组件,并保存为 Prefab。
unity webgl的Dropdown组件怎么用预制体,代码怎么写
要在Unity WebGL中使用Dropdown组件与预制体,需要遵循以下步骤:
1. 在场景中创建一个Dropdown对象。
2. 创建一个预制体,将Dropdown对象作为子对象添加到预制体中。
3. 在代码中,使用PrefabUtility.InstantiatePrefab()方法通过预制体实例化Dropdown对象的实例。
4. 设置Dropdown对象的各项属性,例如选项列表、默认选项等。
5. 将实例化后的Dropdown对象添加到场景中。
下面是一个示例代码:
```csharp
using UnityEngine;
using UnityEngine.UI;
using UnityEditor;
public class DropdownWithPrefab : MonoBehaviour
{
public GameObject dropdownPrefab;
private Dropdown dropdown;
void Start()
{
// 实例化Dropdown预制体
GameObject dropdownObject = (GameObject)PrefabUtility.InstantiatePrefab(dropdownPrefab);
// 获取Dropdown组件
dropdown = dropdownObject.GetComponent<Dropdown>();
// 设置Dropdown选项列表
dropdown.options.Clear();
dropdown.options.Add(new Dropdown.OptionData("Option 1"));
dropdown.options.Add(new Dropdown.OptionData("Option 2"));
dropdown.options.Add(new Dropdown.OptionData("Option 3"));
// 设置默认选项
dropdown.value = 0;
// 将Dropdown添加到场景中
dropdown.transform.SetParent(transform, false);
}
}
```
在这个示例中,我们将Dropdown预制体指定为“dropdownPrefab”变量,并在Start()方法中实例化了一个Dropdown对象。然后,我们设置了Dropdown的选项列表和默认选项,并将它添加到场景中。
注意,我们在使用PrefabUtility.InstantiatePrefab()方法时,需要添加UnityEditor命名空间,因为这个方法只能在Unity编辑器环境下使用。在WebGL构建时,这个方法会被忽略。
希望这个示例能够帮助你使用Dropdown组件与预制体在Unity WebGL中创建下拉列表。
相关推荐
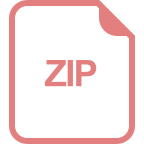
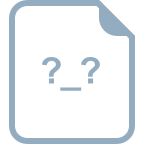












