how to implement minus operation of 16-bit integer in c-language
时间: 2024-05-14 17:17:58 浏览: 117
You can implement the minus operation of 16-bit integer in C-language using the '-' operator. Here is an example:
```c
#include <stdio.h>
int main() {
// Initialize two 16-bit integers
int a = 100;
int b = 50;
// Subtract b from a
int c = a - b;
// Print the result
printf("%d - %d = %d", a, b, c);
return 0;
}
```
In this example, we declare two 16-bit integers `a` and `b`, and then subtract `b` from `a` using the '-' operator. The result is stored in the variable `c` and printed to the console using `printf()`.
相关问题
how to implement negate operation of 16-bit integer
To negate a 16-bit integer, you can use the two's complement method.
Here's how you can implement it in C:
```c
int16_t negate(int16_t x) {
return ~x + 1;
}
```
Explanation:
- The `~` operator performs a bitwise NOT operation on the input value `x`, which inverts all its bits.
- Adding 1 to the inverted value gives us the two's complement of `x`, which is the negated value.
Note that this implementation assumes that `int16_t` is a signed 16-bit integer type. If `int16_t` is an unsigned type, you can't perform a two's complement negation and would need to use a different method.
program a division algorithm without division-instructions for 32-bit integer in C language
One possible way to implement a division algorithm without using division instructions in C language for 32-bit integers is to use repeated subtraction. Here's an example implementation:
```c
unsigned int divide(unsigned int dividend, unsigned int divisor) {
unsigned int quotient = 0;
while (dividend >= divisor) {
dividend -= divisor;
quotient++;
}
return quotient;
}
```
This function takes two unsigned integers, `dividend` and `divisor`, and returns their quotient as an unsigned integer. It repeatedly subtracts `divisor` from `dividend` until `dividend` becomes less than `divisor`. The number of times this subtraction operation is performed is the quotient.
Note that this algorithm does not handle division by zero. Also, since it uses repeated subtraction, it may be slow for large values of `dividend` and `divisor`. There are more efficient algorithms for division, but they typically require more complex implementation.
阅读全文
相关推荐
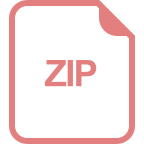
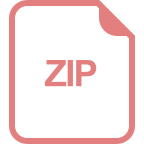
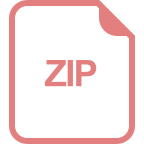
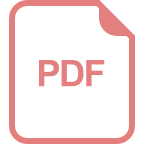
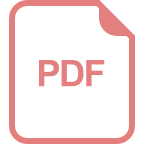
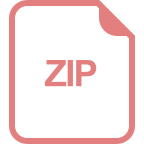
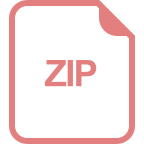
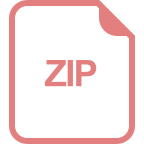
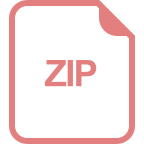
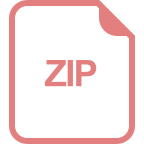
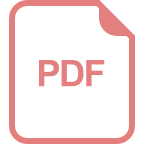
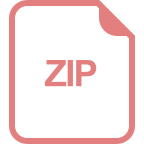
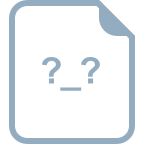
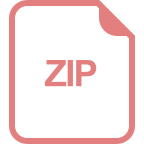
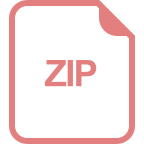
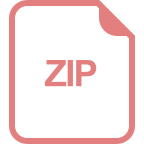
