用python编写一个逻辑回归
时间: 2023-06-30 07:23:56 浏览: 89
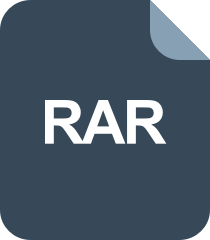
基于python的逻辑回归算法设计与实现
下面是一个简单的示例代码,用 Python 实现逻辑回归模型:
```python
import numpy as np
class LogisticRegression:
def __init__(self, learning_rate=0.01, num_iterations=100000):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
self.weights = None
self.bias = None
def sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def fit(self, X, y):
num_samples, num_features = X.shape
# 初始化权重和偏置
self.weights = np.zeros(num_features)
self.bias = 0
# 梯度下降训练模型
for i in range(self.num_iterations):
# 计算预测值和损失
z = np.dot(X, self.weights) + self.bias
y_pred = self.sigmoid(z)
cost = (-1 / num_samples) * np.sum(y * np.log(y_pred) + (1 - y) * np.log(1 - y_pred))
# 计算梯度
dw = (1 / num_samples) * np.dot(X.T, (y_pred - y))
db = (1 / num_samples) * np.sum(y_pred - y)
# 更新权重和偏置
self.weights -= self.learning_rate * dw
self.bias -= self.learning_rate * db
return self
def predict(self, X):
z = np.dot(X, self.weights) + self.bias
y_pred = self.sigmoid(z)
y_pred_class = [1 if i > 0.5 else 0 for i in y_pred]
return y_pred_class
```
其中,sigmoid 函数用于将预测值转换为概率值,fit 方法用于训练模型,predict 方法用于预测新样本的类别。在训练时,使用梯度下降法更新模型的参数,以最小化损失函数。
阅读全文
相关推荐
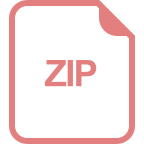
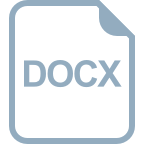

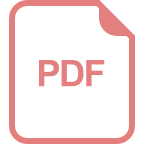

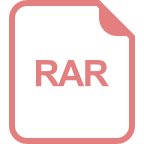
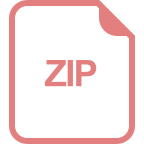
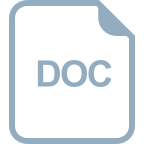
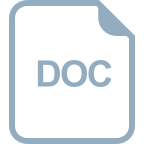
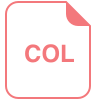






