用Python编程贪吃蛇游戏
时间: 2023-11-05 14:05:35 浏览: 38
由于贪吃蛇游戏比较复杂,需要涉及到图形界面、键盘输入、碰撞检测等多个方面,因此需要使用Python中的pygame模块来实现。
首先,导入pygame模块和其他需要用到的模块:
```python
import pygame
import random
import time
```
然后,设置游戏界面的大小、背景颜色、游戏标题等:
```python
pygame.init()
# 游戏界面大小
width = 600
height = 600
# 创建游戏界面
screen = pygame.display.set_mode((width, height))
# 设置游戏标题
pygame.display.set_caption("贪吃蛇游戏")
# 游戏背景颜色
background_color = (255, 255, 255)
```
接着,定义贪吃蛇的类Snake,包括初始化、移动、吃食物等方法:
```python
class Snake:
def __init__(self):
# 初始蛇的长度为3
self.length = 3
# 初始蛇头位置
self.pos = [[100, 100], [80, 100], [60, 100]]
# 初始蛇移动方向
self.direction = "right"
# 初始化食物位置
self.food = [random.randrange(1, width // 10) * 10, random.randrange(1, height // 10) * 10]
# 贪吃蛇移动
def move(self):
# 判断蛇头移动方向
if self.direction == "right":
self.pos.insert(0, [self.pos[0][0] + 10, self.pos[0][1]])
elif self.direction == "left":
self.pos.insert(0, [self.pos[0][0] - 10, self.pos[0][1]])
elif self.direction == "up":
self.pos.insert(0, [self.pos[0][0], self.pos[0][1] - 10])
elif self.direction == "down":
self.pos.insert(0, [self.pos[0][0], self.pos[0][1] + 10])
# 判断蛇是否吃到食物
if self.pos[0] == self.food:
self.food = [random.randrange(1, width // 10) * 10, random.randrange(1, height // 10) * 10]
self.length += 1
else:
self.pos.pop()
# 贪吃蛇改变移动方向
def change_direction(self, direction):
if direction == "right" and self.direction != "left":
self.direction = "right"
elif direction == "left" and self.direction != "right":
self.direction = "left"
elif direction == "up" and self.direction != "down":
self.direction = "up"
elif direction == "down" and self.direction != "up":
self.direction = "down"
```
然后,定义游戏界面的更新方法,包括绘制蛇、食物、游戏结束等:
```python
# 更新游戏界面
def update_screen():
# 绘制游戏背景
screen.fill(background_color)
# 绘制蛇
for p in snake.pos:
pygame.draw.rect(screen, (0, 255, 0), pygame.Rect(p[0], p[1], 10, 10))
# 绘制食物
pygame.draw.rect(screen, (255, 0, 0), pygame.Rect(snake.food[0], snake.food[1], 10, 10))
# 判断游戏是否结束
if snake.pos[0][0] < 0 or snake.pos[0][0] > width - 10 or snake.pos[0][1] < 0 or snake.pos[0][1] > height - 10:
font = pygame.font.SysFont(None, 50)
text = font.render("Game Over", True, (255, 0, 0))
screen.blit(text, (width // 2 - 100, height // 2 - 25))
pygame.display.update()
time.sleep(2)
pygame.quit()
quit()
# 判断蛇是否撞到自己
for p in snake.pos[1:]:
if snake.pos[0] == p:
font = pygame.font.SysFont(None, 50)
text = font.render("Game Over", True, (255, 0, 0))
screen.blit(text, (width // 2 - 100, height // 2 - 25))
pygame.display.update()
time.sleep(2)
pygame.quit()
quit()
pygame.display.update()
```
最后,实现游戏循环,包括键盘事件的监听、贪吃蛇的移动、游戏界面的更新等:
```python
# 创建贪吃蛇
snake = Snake()
# 游戏循环
while True:
# 监听键盘事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
snake.change_direction("right")
elif event.key == pygame.K_LEFT:
snake.change_direction("left")
elif event.key == pygame.K_UP:
snake.change_direction("up")
elif event.key == pygame.K_DOWN:
snake.change_direction("down")
# 贪吃蛇移动
snake.move()
# 更新游戏界面
update_screen()
# 控制游戏帧率
pygame.time.Clock().tick(10)
```
完整代码如下:
相关推荐
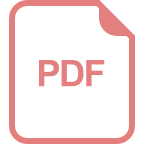
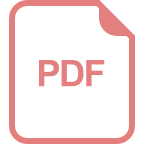
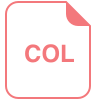
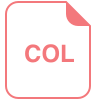
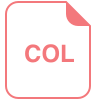
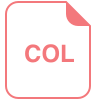
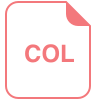









