SemiCircleProgressBar 实现代码 支持渐变色
时间: 2023-06-19 13:06:33 浏览: 83
以下是一个简单的实现 SemiCircleProgressBar 并支持渐变色的示例代码,使用了 Canvas 绘制。
```java
public class SemiCircleProgressBar extends View {
private static final int DEFAULT_START_COLOR = Color.parseColor("#FFC107");
private static final int DEFAULT_END_COLOR = Color.parseColor("#FF5722");
private static final int DEFAULT_ANIM_DURATION = 1000;
private int startColor;
private int endColor;
private int animDuration;
private float progress;
private Paint paint;
private RectF rectF;
public SemiCircleProgressBar(Context context) {
this(context, null);
}
public SemiCircleProgressBar(Context context, AttributeSet attrs) {
super(context, attrs);
TypedArray ta = context.obtainStyledAttributes(attrs, R.styleable.SemiCircleProgressBar);
startColor = ta.getColor(R.styleable.SemiCircleProgressBar_startColor, DEFAULT_START_COLOR);
endColor = ta.getColor(R.styleable.SemiCircleProgressBar_endColor, DEFAULT_END_COLOR);
animDuration = ta.getInteger(R.styleable.SemiCircleProgressBar_animDuration, DEFAULT_ANIM_DURATION);
ta.recycle();
init();
}
private void init() {
paint = new Paint();
paint.setAntiAlias(true);
paint.setStyle(Paint.Style.STROKE);
paint.setStrokeWidth(10);
rectF = new RectF();
}
public void setProgress(float progress) {
this.progress = progress;
invalidate();
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
int width = getWidth();
int height = getHeight();
int centerX = width / 2;
int centerY = height / 2;
int radius = Math.min(centerX, centerY) - 20;
rectF.set(centerX - radius, centerY - radius, centerX + radius, centerY + radius);
LinearGradient gradient = new LinearGradient(0, 0, width, 0, startColor, endColor, Shader.TileMode.CLAMP);
paint.setShader(gradient);
canvas.drawArc(rectF, 180, 180 * progress, false, paint);
}
public void startAnim() {
ValueAnimator animator = ValueAnimator.ofFloat(0, 1);
animator.setDuration(animDuration);
animator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
setProgress((float) animation.getAnimatedValue());
}
});
animator.start();
}
}
```
在布局文件中使用:
```xml
<com.example.SemiCircleProgressBar
android:layout_width="200dp"
android:layout_height="200dp"
app:startColor="#FFC107"
app:endColor="#FF5722"
app:animDuration="1000" />
```
其中,`startColor` 和 `endColor` 分别表示渐变色的起始颜色和结束颜色,`animDuration` 表示动画的时长。可以通过 `setProgress` 方法设置进度,通过 `startAnim` 方法开始动画。
阅读全文
相关推荐

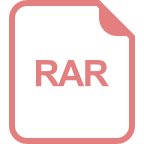
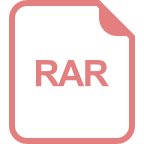
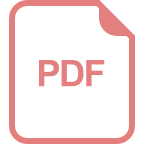
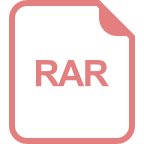
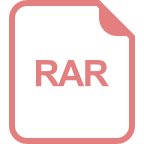
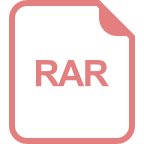
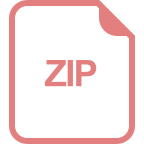
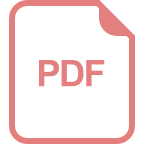
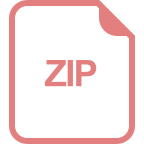
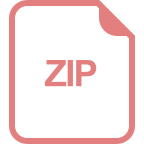
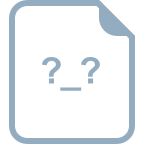
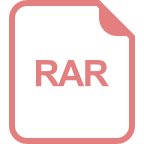
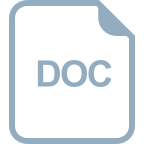
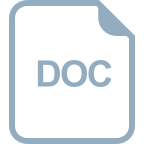