C++编写程序:建立一个10结点的单向链表,每个结点包括学号、姓名、性别、年龄。采用插入排序法对其进行排序,按学号从小到大排列。
时间: 2024-01-13 13:04:03 浏览: 96
好的,我可以为您提供代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 定义链表结点
struct Node {
int id;
string name;
char gender;
int age;
Node* next;
};
// 插入排序
void insertionSort(Node*& head) {
if (head == nullptr || head->next == nullptr) return; // 链表为空或只有一个结点,不需要排序
Node* sorted = head; // 已排序部分的末尾结点
Node* cur = head->next; // 当前待排序结点
sorted->next = nullptr; // 已排序部分的末尾结点的next指针置为nullptr
while (cur != nullptr) {
Node* next = cur->next; // 保存下一个待排序结点,防止链表断裂
if (cur->id < sorted->id) { // 待排序结点的学号小于已排序部分的末尾结点的学号
cur->next = sorted; // 将待排序结点插入已排序部分的开头
sorted = cur;
} else { // 待排序结点的学号大于等于已排序部分的末尾结点的学号
Node* p = sorted;
while (p->next != nullptr && cur->id >= p->next->id) { // 找到待排序结点应插入的位置
p = p->next;
}
cur->next = p->next; // 将待排序结点插入到p结点之后
p->next = cur;
}
cur = next; // 处理下一个待排序结点
}
head = sorted; // 更新链表头
}
// 输出链表
void printList(Node* head) {
while (head != nullptr) {
cout << head->id << " " << head->name << " " << head->gender << " " << head->age << endl;
head = head->next;
}
}
int main() {
// 建立链表
Node* head = new Node{101, "Tom", 'M', 20, nullptr};
Node* p1 = new Node{105, "Lucy", 'F', 19, nullptr};
Node* p2 = new Node{103, "Jack", 'M', 21, nullptr};
Node* p3 = new Node{109, "Lily", 'F', 18, nullptr};
Node* p4 = new Node{107, "Bob", 'M', 22, nullptr};
Node* p5 = new Node{102, "Mary", 'F', 20, nullptr};
Node* p6 = new Node{106, "John", 'M', 19, nullptr};
Node* p7 = new Node{104, "Kate", 'F', 21, nullptr};
Node* p8 = new Node{110, "Mike", 'M', 18, nullptr};
Node* p9 = new Node{108, "Ann", 'F', 22, nullptr};
head->next = p1;
p1->next = p2;
p2->next = p3;
p3->next = p4;
p4->next = p5;
p5->next = p6;
p6->next = p7;
p7->next = p8;
p8->next = p9;
// 对链表进行排序
insertionSort(head);
// 输出排序后的链表
printList(head);
// 释放链表内存
Node* p = head;
while (p != nullptr) {
Node* q = p->next;
delete p;
p = q;
}
return 0;
}
```
以上代码使用插入排序法对单向链表进行排序,按学号从小到大排列。您可以根据需要进行修改。
阅读全文
相关推荐
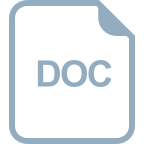
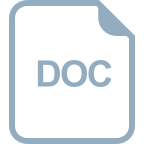
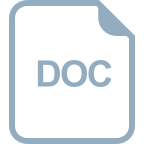
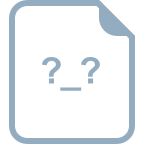
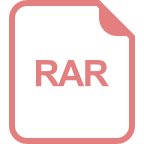
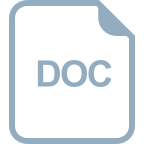




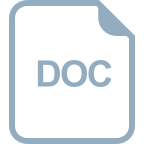
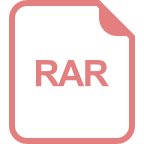
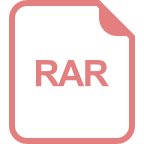
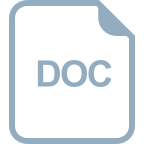
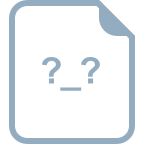