python中字符串的split
时间: 2023-10-19 15:07:49 浏览: 48
在Python中,字符串的split()方法可以将一个字符串按照指定的分隔符分割成多个子字符串,并返回一个包含所有子字符串的列表。
例如,假设有一个字符串s,可以使用以下代码将其按照空格分割成多个子字符串:
```
s = "hello world"
words = s.split()
print(words)
```
运行结果为:
```
['hello', 'world']
```
split()方法也可以接受一个参数,用于指定分隔符。例如,可以使用以下代码将一个逗号分隔的字符串s分割成多个子字符串:
```
s = "apple,banana,orange"
fruits = s.split(",")
print(fruits)
```
运行结果为:
```
['apple', 'banana', 'orange']
```
相关问题
python 分割字符串 split
您好!对于Python中的字符串分割操作,可以使用split()函数。该函数将字符串按照指定的分隔符进行拆分,并返回拆分后的部分作为一个列表。例如:
```python
string = "Hello, World!"
words = string.split(",") # 使用逗号作为分隔符
print(words) # 输出: ['Hello', ' World!']
```
split()函数还可以接受一个可选的参数maxsplit,用于指定最大的拆分次数。如果不提供该参数,默认会将字符串完全拆分。例如:
```python
string = "apple,banana,orange,grape"
fruits = string.split(",", 2) # 拆分成3部分
print(fruits) # 输出: ['apple', 'banana', 'orange,grape']
```
希望能对您有所帮助!如有更多问题,请继续提问。
python 字符串split
在Python中,字符串的split()方法用于将字符串按照指定的分隔符进行分割,并返回一个包含分割后的子字符串的列表。下面是一些关于字符串split()方法的用法示例:
1. 分隔指定的几个字符:
```python
string = "Python is a good language"
content = string.split(" ", 2) # 分隔前2个字符串
print(content)
```
输出结果为:['Python', 'is', 'a good language']
2. 获取某一个切割的字符:
```python
string = "Python is a good language"
content = string.split(" ")[3] # 获取分隔的第四个字符
print(content)
```
输出结果为:'language'
3. 其他规律的指定的分隔符:
```python
string = "Hi:Tom"
content = string.split(":")
print(content)
key = string.split(":")[0]
print(key)
value = string.split(":")[1]
print(value)
string = "abc#def#xyz"
content = string.split("#")
print(content)
content1 = string.split("#")[0]
print(content1)
content2 = string.split("#")[1]
print(content2)
content3 = string.split("#")[2]
print(content3)
```
输出结果为:
```
['Hi', 'Tom']
Hi
Tom
['abc', 'def', 'xyz']
abc
def
xyz
```
希望以上解答能够帮到你!
相关推荐
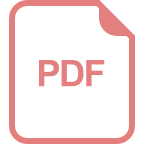
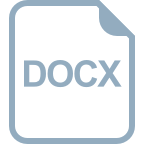
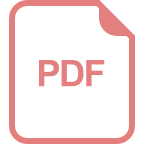












