python找出列表中完全匹配的字符串
时间: 2023-10-15 21:06:56 浏览: 48
可以使用Python中的in关键字来判断一个字符串是否在列表中,如果要找出列表中完全匹配的字符串,可以遍历列表,逐一比较每个字符串是否与目标字符串相等。
以下是一个示例代码:
```python
my_list = ["apple", "banana", "orange", "peach"]
target_str = "orange"
for item in my_list:
if item == target_str:
print("Found a match:", item)
```
输出结果为:
```
Found a match: orange
```
如果要找出所有匹配的字符串,可以将匹配项存储在另一个列表中,如下所示:
```python
my_list = ["apple", "banana", "orange", "peach"]
target_str = "e"
matches = []
for item in my_list:
if item == target_str:
matches.append(item)
print("Found", len(matches), "matches:", matches)
```
输出结果为:
```
Found 0 matches: []
```
在这个例子中,我们搜索的是目标字符串中是否有字符"e"。由于没有完全匹配的字符串,输出结果为空列表。
相关问题
python找出出现次数最多的字符串
### 回答1:
可以使用Python中的Counter模块来统计字符串出现的次数,然后找出出现次数最多的字符串。
具体实现步骤如下:
1. 将字符串转换为列表,方便进行统计。
2. 使用Counter模块统计每个字符串出现的次数,得到一个字典。
3. 使用max函数找出字典中出现次数最多的字符串。
代码示例:
```python
from collections import Counter
s = "hello world, hello python, hello python"
# 将字符串转换为列表
lst = s.split()
# 使用Counter模块统计每个字符串出现的次数
cnt = Counter(lst)
# 使用max函数找出字典中出现次数最多的字符串
most_common_str = max(cnt, key=cnt.get)
print("出现次数最多的字符串是:", most_common_str)
```
输出结果:
```
出现次数最多的字符串是: hello
```
### 回答2:
要找出在一段文本中出现次数最多的字符串,可以使用Python的字典(dictionary)来实现。
首先,可以将文本中的所有单词都存入一个列表中。可以使用split()方法把文本划分成单词,然后用for循环把单词逐个添加到列表中。例如:
text = "This is a sample text with several words. Here are some more words. And here are some more."
words = text.split()
接下来,可以创建一个字典,用来统计每个单词在文本中出现的次数。可以用for循环遍历列表,逐个检查每个单词是否已经在字典中出现过。如果是,就把该单词的计数器加一;如果不是,就把该单词及其计数器加入到字典中。例如:
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
最后,可以用一个for循环遍历字典的所有项,找出出现次数最多的单词。可以用一个变量来记录该单词及其出现次数,然后逐个检查每个单词的出现次数是否大于现有记录的出现次数。如果是,就更新记录。例如:
max_word = ""
max_count = 0
for word in word_count:
if word_count[word] > max_count:
max_word = word
max_count = word_count[word]
最后,变量max_word中就是出现次数最多的单词。在本例中,结果应该是“more”。
### 回答3:
要找出一个字符串列表中出现次数最多的字符串,我们可以使用Python内置的counter类来实现。Counter类是一个字典子类,它可以用来计算一个可迭代对象中元素的出现频率,结果以字典形式返回。
首先,我们需要将字符串列表转换为一个Counter对象。这可以通过使用collections模块中的Counter类来完成。Counter对象可以使用列表的count()方法来计算每个元素出现的次数,然后将计数结果存储在字典中。
接着,我们要找到出现次数最多的字符串。为了找到字典中最大的值,我们可以使用内置的max()函数。max()函数可接受两个参数:一个是要比较的可迭代对象,另一个是一个可选的关键字函数,用于指定比较所用的键。
因此,我们可以使用以下Python代码来找出一个字符串列表中出现次数最多的字符串:
```
from collections import Counter
# 字符串列表
words = ['apple', 'banana', 'cherry', 'apple', 'banana', 'apple', 'cherry']
# 创建Counter对象
counter = Counter(words)
# 找到出现次数最多的字符串
most_common_word = max(counter.keys(), key=lambda x: counter[x])
print("出现次数最多的字符串是:", most_common_word)
```
在这里,Counter对象被创建来计算字符串列表中每个字符串的出现次数。接下来我们使用max()函数来找到最大的计数值,并取出对应的字符串。最后,我们打印出找到的字符串。
以上便是使用Python找出出现次数最多的字符串的方法。利用Python中的Counter对象,我们可以方便地计算列表中元素的出现频率,并快速找到最常出现的字符串。
python找出给定字符串中相同单词
可以使用Python的split()函数将字符串按照空格分割成单词列表,然后使用Python的Counter类统计每个单词出现的次数,最后筛选出出现次数大于1的单词,即为给定字符串中相同的单词。以下是示例代码:
```python
from collections import Counter
def find_same_words(s):
words = s.split()
counter = Counter(words)
return [w for w in counter if counter[w] > 1]
s = "hello world hello python world python python"
print(find_same_words(s)) # 输出 ['hello', 'world', 'python']
```
这段代码会输出给定字符串中出现次数大于1的单词列表,即 `['hello', 'world', 'python']`。
相关推荐
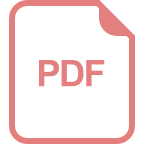












