使用JFrame创建登录窗口(可参考下图或自行设计布局) 建立用户表user,当点击登录按钮时,对输入的用户名为和密码进行验 证: 当输入的用户和密码与user表中某一条记录相匹配时,弹出消息对话框,提示信息为:“用户名和密码正确”,否则,提示信息为:“用户名或密码错误”。 点击重置按钮时: 用户名文本框和密码文本框的内容被清空。
时间: 2024-03-13 16:48:03 浏览: 121
好的,这是一个简单的Java Swing程序,需要用到JFrame、JPanel、JLabel、JTextField、JPasswordField、JButton、JOptionPane等组件。下面是代码实现:
```java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class LoginFrame extends JFrame implements ActionListener {
private JPanel panel;
private JLabel userLabel, passwordLabel;
private JTextField userText;
private JPasswordField passwordText;
private JButton loginButton, resetButton;
public LoginFrame() {
panel = new JPanel();
userLabel = new JLabel("用户名:");
passwordLabel = new JLabel("密 码:");
userText = new JTextField(20);
passwordText = new JPasswordField(20);
loginButton = new JButton("登录");
resetButton = new JButton("重置");
panel.add(userLabel);
panel.add(userText);
panel.add(passwordLabel);
panel.add(passwordText);
panel.add(loginButton);
panel.add(resetButton);
loginButton.addActionListener(this);
resetButton.addActionListener(this);
this.add(panel);
this.setTitle("登录窗口");
this.setSize(300, 200);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
public static void main(String args[]) {
new LoginFrame();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == loginButton) {
String user = userText.getText();
String password = new String(passwordText.getPassword());
if (user.equals("") || password.equals("")) {
JOptionPane.showMessageDialog(null, "用户名或密码不能为空!");
return;
}
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/test";
String username = "root";
String pwd = "123456";
conn = DriverManager.getConnection(url, username, pwd);
String sql = "select * from user where username=? and password=?";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, user);
ps.setString(2, password);
ResultSet rs = ps.executeQuery();
if (rs.next()) {
JOptionPane.showMessageDialog(null, "用户名和密码正确!");
} else {
JOptionPane.showMessageDialog(null, "用户名或密码错误!");
}
rs.close();
ps.close();
} catch (Exception ex) {
ex.printStackTrace();
} finally {
try {
if (conn != null) {
conn.close();
}
} catch (Exception ex) {
ex.printStackTrace();
}
}
} else if (e.getSource() == resetButton) {
userText.setText("");
passwordText.setText("");
}
}
}
```
需要注意的是,上面的程序使用了MySQL数据库,需要先安装MySQL,并且创建一个名为test的数据库,在test数据库中创建一个名为user的用户表,表结构如下:
```sql
CREATE TABLE `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(255) NOT NULL,
`password` varchar(255) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
然后向user表中插入一些测试数据,例如:
```sql
INSERT INTO `user` (`id`, `username`, `password`) VALUES
(1, 'admin', '123456'),
(2, 'tom', 'abc123'),
(3, 'jack', 'password');
```
这样就可以运行上面的Java程序进行测试了。
阅读全文
相关推荐
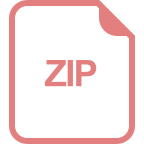
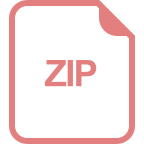
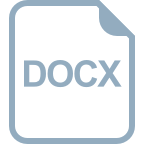
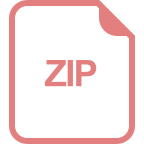
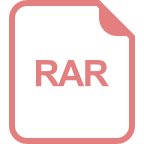
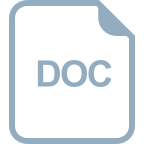
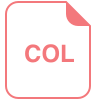
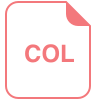
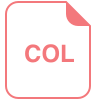
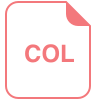
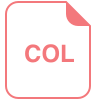
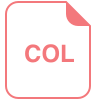
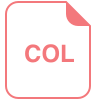
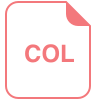
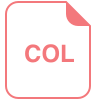
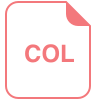



