帮我用C语言写个定时开关的
时间: 2023-09-21 08:06:11 浏览: 172
### 回答1:
可以的,以下是一个简单的定时开关的 C 语言代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int hour, minute, second, duration;
time_t start_time, end_time;
printf("请输入定时开关的时间(小时 分钟 秒):");
scanf("%d %d %d", &hour, &minute, &second);
printf("请输入定时开关的持续时间(秒):");
scanf("%d", &duration);
// 获取当前时间
time(&start_time);
// 计算定时开关的结束时间
struct tm *end_tm = localtime(&start_time);
end_tm->tm_hour = hour;
end_tm->tm_min = minute;
end_tm->tm_sec = second;
end_time = mktime(end_tm);
// 等待定时开关的时间到达
while (difftime(end_time, start_time) > ) {
time(&start_time);
}
// 执行定时开关的操作
printf("定时开关已经开启!\n");
// 等待定时开关的持续时间
sleep(duration);
// 关闭定时开关
printf("定时开关已经关闭!\n");
return ;
}
### 回答2:
当然可以帮您用C语言编写一个定时开关的程序。
首先,我们需要使用C语言中的时间函数库来实现定时功能,其中包括<time.h>和<unistd.h>。
以下是一个示例程序,该程序具有开关功能,并在指定的时间段内打开或关闭开关。
```c
#include <stdio.h>
#include <time.h>
#include <unistd.h>
int main() {
time_t currentTime;
struct tm *localTime;
int hour, minute;
int switchState = 0; // 开关状态,0表示关闭,1表示打开
// 获取当前时间
currentTime = time(NULL);
localTime = localtime(¤tTime);
// 获取用户输入的定时时间
printf("请输入定时开关的时间(小时 分钟,24小时制):");
scanf("%d %d", &hour, &minute);
// 计算定时时间的时间戳
struct tm scheduledTime = *localTime;
scheduledTime.tm_hour = hour;
scheduledTime.tm_min = minute;
time_t scheduledTimestamp = mktime(&scheduledTime);
// 比较当前时间与定时时间
if (currentTime >= scheduledTimestamp) {
// 如果当前时间晚于等于定时时间,则开关打开
switchState = 1;
}
// 不断检查当前时间,并更新开关状态
while (1) {
currentTime = time(NULL);
localTime = localtime(¤tTime);
// 比较当前时间与定时时间
if (currentTime >= scheduledTimestamp && switchState == 0) {
// 如果当前时间晚于等于定时时间且开关为关闭状态,则打开开关
printf("现在是定时开关时间,已打开开关!\n");
switchState = 1;
} else if (currentTime < scheduledTimestamp && switchState == 1) {
// 如果当前时间早于定时时间且开关为打开状态,则关闭开关
printf("定时开关时间已过,已关闭开关!\n");
switchState = 0;
}
// 休眠1秒,继续检查时间
sleep(1);
}
return 0;
}
```
上述程序会要求您输入定时开关的时间(小时和分钟,24小时制),然后不断检查当前时间和定时时间的关系,并输出相应的开关状态信息。
请注意,此程序是一个简单的示例,您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
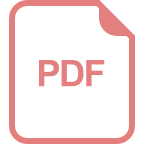
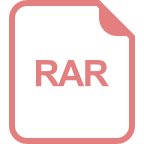
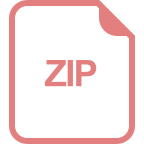
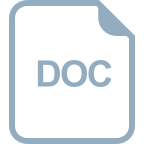
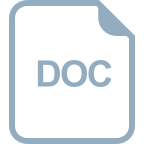











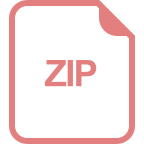
