opencv图像中频域滤波去除噪声,使用c++代码
时间: 2023-11-14 10:13:01 浏览: 130
以下是使用OpenCV C++实现频域滤波去除噪声的示例代码。首先,我们将读取一张图像并添加高斯噪声。然后,我们将对图像进行频域滤波以去除噪声。
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像
Mat image = imread("image.jpg", IMREAD_GRAYSCALE);
// 添加高斯噪声
Mat noise;
randn(noise, 0, 50);
Mat noisy_image = image + noise;
// 显示原始图像和加噪声后的图像
imshow("Original Image", image);
imshow("Noisy Image", noisy_image);
// 计算傅里叶变换
Mat complex_image;
Mat padded_image;
int m = getOptimalDFTSize(noisy_image.rows);
int n = getOptimalDFTSize(noisy_image.cols);
copyMakeBorder(noisy_image, padded_image, 0, m - noisy_image.rows, 0, n - noisy_image.cols, BORDER_CONSTANT, Scalar::all(0));
Mat planes[] = { Mat_<float>(padded_image), Mat::zeros(padded_image.size(), CV_32F) };
merge(planes, 2, complex_image);
dft(complex_image, complex_image);
// 创建低通滤波器
Mat low_pass_filter = Mat::zeros(complex_image.size(), CV_32F);
circle(low_pass_filter, Point(low_pass_filter.cols / 2, low_pass_filter.rows / 2), 50, Scalar(1), -1);
// 应用低通滤波器
Mat filtered_image;
mulSpectrums(complex_image, low_pass_filter, complex_image, 0);
idft(complex_image, filtered_image, DFT_SCALE | DFT_REAL_OUTPUT);
// 显示过滤后的图像
imshow("Filtered Image", filtered_image);
waitKey(0);
return 0;
}
```
在上面的代码中,我们首先读取图像并添加高斯噪声。然后,我们使用OpenCV的DFT函数计算图像的傅里叶变换,并创建一个低通滤波器。通过使用mulSpectrums函数将图像的傅里叶变换和滤波器相乘,我们可以将高频噪声过滤掉。最后,我们使用IDFT函数计算过滤后的图像。
在运行上面的代码后,您应该能够看到原始图像、加噪声的图像和过滤后的图像。过滤后的图像中的噪声应该比加噪声的图像中的噪声要少。
阅读全文
相关推荐








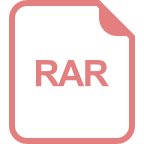










