swiftui为什么控件内无法使用DispatchQueue
时间: 2023-12-06 10:02:46 浏览: 39
在SwiftUI中,你可以使用异步任务来执行后台工作,例如使用`async`和`await`关键字。这些关键字使得在异步任务中使用`DispatchQueue`变得不必要。
异步任务可以让你在后台执行繁重的任务,而不会影响UI的响应性。在异步任务中,你可以执行任何任务,包括使用`DispatchQueue`。
如果您仍然想在SwiftUI中使用`DispatchQueue`,则可以将它们包装在异步任务中执行。示例代码如下:
```swift
struct ContentView: View {
var body: some View {
Button("Perform task") {
Task {
await performTask()
}
}
}
func performTask() async {
await DispatchQueue.global().async {
// 在后台线程执行的代码
}
}
}
```
在此示例中,我们使用异步任务来执行`performTask()`函数,该函数本身包装了`DispatchQueue.global().async`,以便在后台线程执行代码。
相关问题
ios自定义分段控件_使用swiftui自定义ios分段控件
使用SwiftUI自定义iOS分段控件可以使用以下步骤:
1. 创建一个新的SwiftUI View,例如SegmentedControl。
2. 在该视图中,创建一个自定义的SegmentedControl控件。可以使用HStack和Button等SwiftUI组件来创建。
3. 创建一个可绑定的属性来表示选中的选项。这可以是一个字符串或枚举类型。
4. 在每个按钮上添加一个操作,以便在用户点击时更新选定的选项。
5. 添加一些样式和布局选项,例如设置字体和颜色,以及调整大小和间距。
示例代码如下:
```swift
struct SegmentedControl: View {
@Binding var selection: Int
let options = ["Option 1", "Option 2", "Option 3"]
var body: some View {
HStack {
ForEach(0..<options.count) { index in
Button(action: {
self.selection = index
}) {
Text(self.options[index])
.font(.headline)
.foregroundColor(self.selection == index ? .white : .gray)
.padding()
}
.background(self.selection == index ? Color.blue : Color.clear)
.cornerRadius(10)
}
}
.padding()
}
}
```
在使用该控件时,只需将选项绑定到一个可变的属性即可:
```swift
struct ContentView: View {
@State var selection = 0
var body: some View {
VStack {
SegmentedControl(selection: $selection)
Text("Selected Option: \(selection)")
}
}
}
```
这个自定义的SegmentedControl将会显示三个选项,每个选项都是一个按钮,用户点击按钮时将更新选择的值。选中的选项将会呈现为蓝色背景和白色文本。
swiftui放大缩小控件
在 SwiftUI 中,你可以使用 `scaleEffect` 修饰符来放大或缩小视图。下面是一个例子:
```swift
struct ContentView: View {
@State private var scale: CGFloat = 1.0
var body: some View {
VStack {
Image(systemName: "star.fill")
.font(.system(size: 100))
.scaleEffect(scale)
.gesture(MagnificationGesture()
.onChanged { value in
self.scale = value.magnitude
}
)
Text("Pinch to zoom")
.font(.headline)
}
}
}
```
在上面的代码中,我们创建了一个 `Image` 视图,并为其添加了 `scaleEffect(scale)` 修饰符。`scale` 是一个 `@State` 属性,用于控制视图的缩放比例。我们还将 `MagnificationGesture` 添加到图像上,以允许用户使用捏合手势来缩放图像。在 `onChanged` 闭包中,我们更新了 `scale` 属性,以便图像的缩放比例可以反映用户手势的变化。
当你运行上面的代码,你会看到一个可以缩放的星星图标。你可以使用捏合手势来放大或缩小图标。
相关推荐
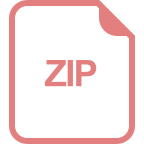
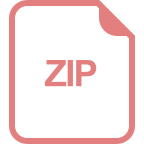












