用java写一个全球通计费程序
时间: 2023-12-02 16:04:05 浏览: 65
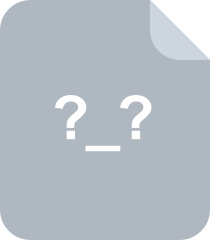
Rust 学习教程(入门到实践)
好的,我可以为你提供一个基本的Java代码框架,你可以根据自己的需求进行修改和完善。以下是一个简单的全球通计费程序的代码示例:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
interface Plan {
double calculateFee(int minutes);
}
class SilverPlan implements Plan {
public double calculateFee(int minutes) {
double baseFee = 29.95;
double additionalFee = 0.45 * (minutes - 500);
if (additionalFee < 0) {
additionalFee = 0;
}
return baseFee + additionalFee;
}
}
class GoldPlan implements Plan {
public double calculateFee(int minutes) {
double baseFee = 49.95;
double additionalFee = 0.25 * (minutes - 1000);
if (additionalFee < 0) {
additionalFee = 0;
}
return baseFee + additionalFee;
}
}
class BillingSystem implements ActionListener {
private JFrame frame;
private JTextField phoneNumberField;
private JTextField minutesField;
private JComboBox<String> planComboBox;
private JLabel resultLabel;
public BillingSystem() {
frame = new JFrame("全球通计费程序");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
frame.setLayout(new GridLayout(4, 2));
frame.add(new JLabel("电话号码:"));
phoneNumberField = new JTextField(10);
frame.add(phoneNumberField);
frame.add(new JLabel("通话分钟数:"));
minutesField = new JTextField(10);
frame.add(minutesField);
frame.add(new JLabel("套餐类型:"));
String[] planTypes = {"银卡", "金卡"};
planComboBox = new JComboBox<String>(planTypes);
frame.add(planComboBox);
JButton calculateButton = new JButton("计算");
calculateButton.addActionListener(this);
frame.add(calculateButton);
resultLabel = new JLabel();
frame.add(resultLabel);
frame.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String phoneNumber = phoneNumberField.getText();
int minutes = Integer.parseInt(minutesField.getText());
String planType = (String)planComboBox.getSelectedItem();
Plan plan;
if (planType.equals("银卡")) {
plan = new SilverPlan();
} else {
plan = new GoldPlan();
}
double fee = plan.calculateFee(minutes);
String result = String.format("电话号码:%s,话费:%f 元", phoneNumber, fee);
resultLabel.setText(result);
}
public static void main(String[] args) {
BillingSystem billingSystem = new BillingSystem();
}
}
```
这个程序使用了Java的面向对象编程思想,通过接口和多个子类实现了不同套餐类型的话费计算。在图形用户界面中,用户可以输入电话号码、通话分钟数和套餐类型,并点击“计算”按钮进行计费。计费结果会显示在界面上。
需要注意的是,这个程序只是一个简单的示例,没有考虑到很多实际情况,比如说不同地区的资费标准、套餐类型的变动等。如果你要开发一个真正的全球通计费程序,需要对这些因素进行更复杂的考虑和处理。
阅读全文
相关推荐
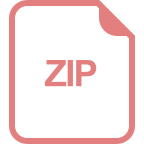
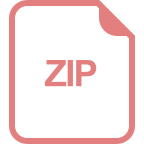
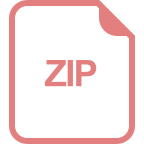
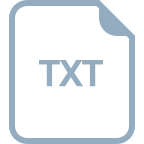
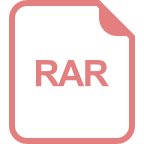
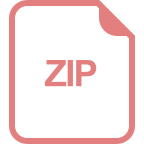
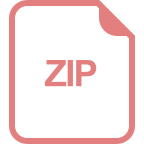