c++判断文件是否为空
时间: 2023-08-06 18:02:51 浏览: 131
可以使用C++中的文件流来判断文件是否为空。具体操作如下:
```c++
#include <fstream>
using namespace std;
int main()
{
ifstream file("filename.txt"); //打开文件
if (file.peek() == ifstream::traits_type::eof()) //判断文件是否为空
{
//文件为空
}
else
{
//文件不为空
}
file.close(); //关闭文件
return 0;
}
```
在上述代码中,我们使用`ifstream`类来打开文件,并使用`peek()`函数来读取文件的第一个字符。如果文件为空,则`peek()`函数返回`EOF`(-1),否则返回文件的第一个字符。因此,我们可以通过判断`peek()`函数的返回值是否等于`EOF`来判断文件是否为空。
相关问题
C++,判断一个文件是否为空
在 C++ 中,你可以使用 `std::ifstream` 来打开文件并判断文件是否为空。以下是一个示例代码:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream file("filename");
if (file.peek() == std::ifstream::traits_type::eof()) {
cout << "File is empty" << endl;
} else {
cout << "File is not empty" << endl;
}
file.close();
return 0;
}
```
其中,`std::ifstream::traits_type::eof()` 可以判断文件是否已经到达文件末尾(即文件是否为空)。如果文件为空,则 `file.peek()` 返回值为 `std::ifstream::traits_type::eof()`。
C++中如何判断一个文件是否为空
在C++中,判断一个文件是否为空,通常需要先打开文件,然后读取一些内容到缓冲区,如果读取的内容长度为0,那么可以认为文件是空的。这里是一个简单的示例:
```cpp
#include <fstream>
#include <vector>
bool isFileEmpty(const std::string& filename) {
std::ifstream file(filename);
if (!file) {
// 文件不存在或者无法打开
return true;
}
// 创建一个小的缓冲区来读取数据
char buffer[1];
file.read(buffer, 1);
// 如果读取失败(如EOF),则返回true表示文件为空
if (file.gcount() == 0 || !file)
return true;
// 文件非空,关闭并返回false
file.close();
return false;
}
```
在这个函数中,我们尝试读取一个字符到`buffer`,如果`gcount()`返回0(即没有读取到数据),或者`!file`(文件流结束),则说明文件是空的。
阅读全文
相关推荐
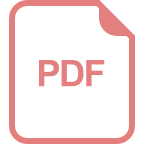











