我需要蜂窝拖动缩放的c语言代码
时间: 2023-10-15 09:02:12 浏览: 69
以下是一个简单的基于 OpenGL 的蜂窝拖动缩放的 C 语言代码:
```c
#include <GL/glut.h>
// 定义屏幕宽度和高度
const int SCREEN_WIDTH = 640;
const int SCREEN_HEIGHT = 480;
// 定义蜂窝大小和缩放因子
const int CELL_SIZE = 10;
float zoom = 1.0;
// 定义蜂窝类
typedef struct {
int x;
int y;
int state;
} Cell;
// 定义蜂窝画布类
typedef struct {
int width;
int height;
Cell cells[100][100];
} Canvas;
Canvas canvas;
// 初始化蜂窝画布
void init_canvas() {
canvas.width = SCREEN_WIDTH / CELL_SIZE;
canvas.height = SCREEN_HEIGHT / CELL_SIZE;
for (int y = 0; y < canvas.height; y++) {
for (int x = 0; x < canvas.width; x++) {
canvas.cells[x][y].x = x;
canvas.cells[x][y].y = y;
canvas.cells[x][y].state = 0;
}
}
}
// 绘制蜂窝
void draw_cells() {
for (int y = 0; y < canvas.height; y++) {
for (int x = 0; x < canvas.width; x++) {
Cell cell = canvas.cells[x][y];
if (cell.state == 1) {
glColor3f(1.0, 0.0, 0.0);
} else {
glColor3f(0.0, 0.0, 0.0);
}
glBegin(GL_QUADS);
glVertex2f((cell.x - canvas.width / 2) * CELL_SIZE * zoom, (cell.y - canvas.height / 2) * CELL_SIZE * zoom);
glVertex2f((cell.x - canvas.width / 2) * CELL_SIZE * zoom + CELL_SIZE * zoom, (cell.y - canvas.height / 2) * CELL_SIZE * zoom);
glVertex2f((cell.x - canvas.width / 2) * CELL_SIZE * zoom + CELL_SIZE * zoom, (cell.y - canvas.height / 2) * CELL_SIZE * zoom + CELL_SIZE * zoom);
glVertex2f((cell.x - canvas.width / 2) * CELL_SIZE * zoom, (cell.y - canvas.height / 2) * CELL_SIZE * zoom + CELL_SIZE * zoom);
glEnd();
}
}
}
// 处理窗口大小变化事件
void reshape(int w, int h) {
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(-w / 2, w / 2, -h / 2, h / 2, -1, 1);
glMatrixMode(GL_MODELVIEW);
}
// 处理鼠标事件
void mouse(int button, int state, int x, int y) {
if (button == GLUT_LEFT_BUTTON && state == GLUT_DOWN) {
int cell_x = (x - SCREEN_WIDTH / 2) / CELL_SIZE / zoom + canvas.width / 2;
int cell_y = (SCREEN_HEIGHT / 2 - y) / CELL_SIZE / zoom + canvas.height / 2;
if (cell_x >= 0 && cell_x < canvas.width && cell_y >= 0 && cell_y < canvas.height) {
canvas.cells[cell_x][cell_y].state = 1 - canvas.cells[cell_x][cell_y].state;
}
} else if (button == GLUT_RIGHT_BUTTON && state == GLUT_DOWN) {
int cell_x = (x - SCREEN_WIDTH / 2) / CELL_SIZE / zoom + canvas.width / 2;
int cell_y = (SCREEN_HEIGHT / 2 - y) / CELL_SIZE / zoom + canvas.height / 2;
if (cell_x >= 0 && cell_x < canvas.width && cell_y >= 0 && cell_y < canvas.height) {
canvas.cells[cell_x][cell_y].state = 0;
}
} else if (button == GLUT_MIDDLE_BUTTON && state == GLUT_DOWN) {
zoom += 0.1;
} else if (button == GLUT_MIDDLE_BUTTON && state == GLUT_UP) {
zoom -= 0.1;
}
}
// 处理键盘事件
void keyboard(unsigned char key, int x, int y) {
if (key == 'q' || key == 'Q' || key == 27) {
exit(0);
}
}
// 定时更新蜂窝状态
void timer(int value) {
// 更新蜂窝状态
// ...
// 重新绘制
glutPostRedisplay();
// 设置下一次更新时间
glutTimerFunc(100, timer, 0);
}
// 绘制回调函数
void display() {
glClear(GL_COLOR_BUFFER_BIT);
draw_cells();
glutSwapBuffers();
}
int main(int argc, char **argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB);
glutInitWindowSize(SCREEN_WIDTH, SCREEN_HEIGHT);
glutCreateWindow("Cellular Automata");
init_canvas();
glutReshapeFunc(reshape);
glutMouseFunc(mouse);
glutKeyboardFunc(keyboard);
glutDisplayFunc(display);
glutTimerFunc(100, timer, 0);
glutMainLoop();
return 0;
}
```
这个代码可以实现蜂窝的拖动缩放,您可以根据需要进行修改和扩展。
阅读全文
相关推荐
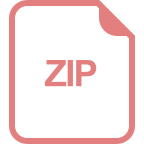
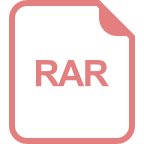
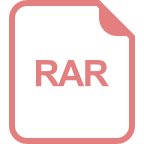















