用java语言完成以下代码:const int m=3;int n=0; void incr ( char id){ int i; for (i=1;i<=m; i++){ n=n+1; cout<<id<<": "<<i<<"@n="<<n<<endl; } } void main (){ cobegin { incr ( 'A') ;incr ( 'B') ;incr ( 'c') ; } cout<<"This sum is: "<<n<<endl;
时间: 2023-06-19 19:07:27 浏览: 151
把C++代码翻译成Java代码如下:
```java
public class Main {
static final int m = 3;
static int n = 0;
public static void incr(char id) {
for (int i = 1; i <= m; i++) {
n = n + 1;
System.out.println(id + ": " + i + "@n=" + n);
}
}
public static void main(String[] args) {
new Thread(() -> incr('A')).start();
new Thread(() -> incr('B')).start();
new Thread(() -> incr('C')).start();
System.out.println("This sum is: " + n);
}
}
```
其中`cobegin`和`cout`是C++的关键字,Java中没有对应的语法,需要用其他方式替换。在这里,我们使用Java 8的Lambda表达式和`System.out.println`来替换。同时,我们在`main`方法里启动了三个线程。
相关问题
class Student { private: int Id, score; char name[9]; public: Student(int id = 10000, const char* n = NULL, int s = 0); int GetId() const; const char* GetName() const; int GetScore() const; void Set(int id = 99999, const char* n = "NONAME", int s = 100); void Show() const; }; Student::Student( int id,const char* n,int s ) { Id=id; score=s; strcpy(name,n); } int Student::GetId() const { return Id; } int Student::GetScore() const { return score; } const char* Student::GetName() const { return name; } void Student::Set( int id,const char* n,int s ) { Id=id; score=s; strcpy(name,n); } void Student::Show() const { cout<<Id<<"-"<<name<<"-"<<score<<endl;}这段代码有何问题
这段代码没有明显的语法错误,但是存在一些潜在问题:
1. char数组name[9]可能无法容纳超过8个字符的字符串,如果传入的n参数长度大于8,会出现问题。
2. 在构造函数中,如果传入的n为NULL指针,会导致strcpy函数出错。
3. 在Set函数中,如果传入的n为NULL指针,会导致strcpy函数出错。
4. 在Show函数中,没有考虑name中存在空格的情况,输出可能会出现问题。
5. 没有对传入的id和score进行有效范围的检查,可能会出现不合法的值。
#include<iostream> using namespace std; class Log { public: const int Erroring = 0; //错误 const int Warning = 1; //警告 const int Info = 2; private: //标记私有 int m_loglevel; public: //标记公开 void SetLevel(int level) { m_loglevel = level; } void Error(const char* a) { if (m_loglevel >= Erroring){ std::cout << "[Error]: " << a << std::endl; } } void Warn(const char* a) { if (m_loglevel >= Warning) { std::cout << "[Warning]: " << a << std::endl; } } void Information(const char* a) { if (m_loglevel >= Info) { std::cout << "[Information]: " << a << std::endl; } } Log() = default; }; int main() { Log log; log.SetLevel(log.Warning);//打印的程度 log.Warn("Hello 1"); log.Error("Hello 2"); log.Information("Hello 3"); std::cin.get(); }
这段代码定义了一个名为 Log 的类,用于记录不同级别的日志消息。代码中的主要部分如下:
```cpp
class Log {
public:
const int Erroring = 0; //错误
const int Warning = 1; //警告
const int Info = 2;
private:
int m_loglevel; //日志级别
public:
void SetLevel(int level) {
m_loglevel = level;
}
void Error(const char* a) {
if (m_loglevel >= Erroring) {
std::cout << "[Error]: " << a << std::endl;
}
}
void Warn(const char* a) {
if (m_loglevel >= Warning) {
std::cout << "[Warning]: " << a << std::endl;
}
}
void Information(const char* a) {
if (m_loglevel >= Info) {
std::cout << "[Information]: " << a << std::endl;
}
}
Log() = default;
};
```
在 `main()` 函数中,我们创建了一个名为 `log` 的 `Log` 类对象,然后通过调用 `SetLevel()` 方法来设置日志级别。接下来,我们使用 `Warn()`、`Error()` 和 `Information()` 方法分别输出不同级别的日志消息。
运行这段代码将输出以下内容:
```
[Warning]: Hello 1
[Error]: Hello 2
```
由于日志级别设置为 `Warning`,所以只有警告级别和更高级别的日志消息被打印出来。
希望这能帮助到你!如果你有任何其他问题,请随时提问。
阅读全文
相关推荐
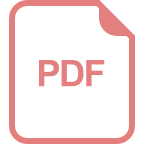
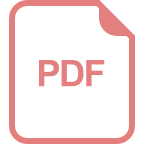













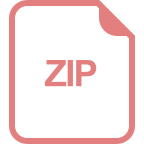
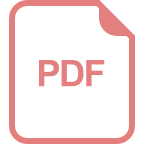