python写一个定时打开绝对路径的文件请提供例子代码
时间: 2023-09-11 22:05:13 浏览: 119
### 回答1:
示例代码如下:import schedule
import time
import osdef open_file():
os.startfile(r"C:\absolute\path\to\file.txt")schedule.every().day.at("10:30").do(open_file)while True:
schedule.run_pending()
time.sleep(1)
### 回答2:
以下是使用Python编写的定时打开绝对路径文件的示例代码:
```python
import os
import time
def open_file_at_time(file_path, hour, minute):
while True:
current_time = time.localtime()
if current_time.tm_hour == hour and current_time.tm_min == minute:
try:
os.startfile(file_path) # 使用默认程序打开文件
break
except OSError:
print("无法打开文件")
time.sleep(60) # 每隔60秒检查一次时间
# 示例:在每天的14点30分定时打开文件
file_path = "C:/example/file.txt"
hour = 14
minute = 30
open_file_at_time(file_path, hour, minute)
```
上述代码中,`open_file_at_time` 函数接收三个参数,分别是文件路径`file_path`、小时`hour`和分钟`minute`。函数将持续进行时间的检查,当当前时间与指定的小时和分钟匹配时,将尝试使用默认程序打开文件,否则每隔60秒重新检查一次时间。
在示例代码中,假设需要在每天的14点30分打开路径为`C:/example/file.txt`的文件。可以根据实际需求修改文件路径、小时和分钟来定时打开指定文件。
### 回答3:
下面是一个使用Python定时打开绝对路径文件的例子代码:
```python
import os
import time
def open_file(file_path):
# 检查文件是否存在
if os.path.exists(file_path):
# 使用系统默认程序打开文件
os.startfile(file_path)
print("成功打开文件:", file_path)
else:
print("文件不存在:", file_path)
def schedule_open_file(file_path, hour, minute):
while True:
current_time = time.localtime(time.time())
# 检查当前时间是否达到预定的打开时间
if current_time.tm_hour == hour and current_time.tm_min == minute:
open_file(file_path)
break
# 等待1分钟
time.sleep(60)
if __name__ == '__main__':
file_path = "C:\\path\\to\\file.txt" # 请替换为实际的文件路径
schedule_open_file(file_path, 9, 0) # 每天9:00打开文件
```
以上代码首先定义了一个`open_file`函数,用于检查文件是否存在,并通过系统默认程序打开文件。然后定义了一个`schedule_open_file`函数,用于定时打开文件。该函数会循环检查当前时间是否达到预定的打开时间,如果达到则调用`open_file`函数打开文件。如果文件不存在,则会在控制台输出相应信息。
在`main`函数中,我们指定了文件的绝对路径`file_path`,并调用`schedule_open_file`函数来设置定时打开文件的时间。以上例子中设置的是每天9点0分打开文件,你可以根据需要自行修改。需要确保文件路径是正确的,并且系统有默认程序关联该文件类型,以便成功打开文件。
阅读全文
相关推荐
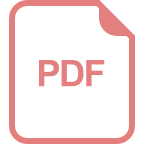
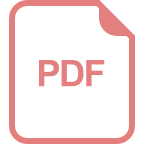
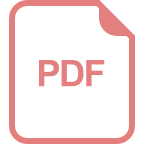
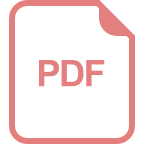
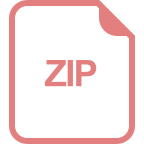
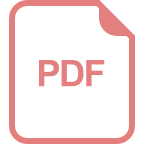
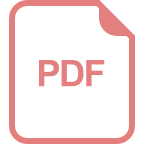
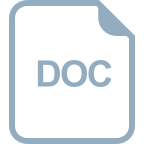
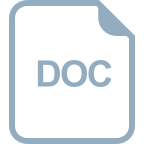
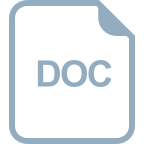
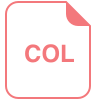
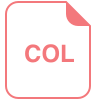
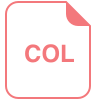






