编写一段dwrr rtl代码
时间: 2024-05-02 12:16:52 浏览: 20
DWRR(Deficit Weighted Round Robin)是一种调度算法,可以用来平衡带宽分配和流量公平性。下面是一段DWRR的RTL代码示例:
```verilog
module dwrr
#(
parameter DWRR_DEPTH = 16, // DWRR队列深度
parameter DWRR_WIDTH = 8, // DWRR队列宽度
parameter DWRR_WEIGHT = 1 // DWRR队列权重
)
(
input clk, // 时钟信号
input rst, // 复位信号
input [DWRR_DEPTH-1:0][DWRR_WIDTH-1:0] input_q, // DWRR队列输入
input [DWRR_DEPTH-1:0] input_valid, // DWRR队列输入有效信号
output reg [DWRR_WIDTH-1:0] output_q, // DWRR队列输出
output reg output_valid // DWRR队列输出有效信号
);
reg [DWRR_DEPTH-1:0] deficit;
reg [DWRR_DEPTH-1:0] current_weight;
reg [DWRR_DEPTH-1:0] next_weight;
reg [DWRR_DEPTH-1:0] next_deficit;
reg [DWRR_DEPTH-1:0] round_robin_pointer;
reg [DWRR_DEPTH-1:0] current_pointer;
reg [DWRR_DEPTH-1:0] next_pointer;
always @(posedge clk or negedge rst) begin
if(!rst) begin
deficit <= 0;
current_weight <= 0;
next_weight <= 0;
next_deficit <= 0;
round_robin_pointer <= 0;
current_pointer <= 0;
next_pointer <= 0;
output_valid <= 0;
end else begin
current_pointer <= next_pointer;
current_weight <= next_weight;
deficit <= next_deficit;
if(input_valid[current_pointer]) begin
// 计算当前队列的权重和赤字
current_weight <= DWRR_WEIGHT;
deficit <= deficit - current_weight;
// 如果当前队列的赤字已经小于0,则输出该队列
if(deficit < 0) begin
output_q <= input_q[current_pointer];
output_valid <= 1;
next_pointer <= (current_pointer + 1) % DWRR_DEPTH;
next_weight <= current_weight;
next_deficit <= deficit + next_weight;
end else begin
// 否则,指针移动到下一个队列
next_pointer <= (current_pointer + 1) % DWRR_DEPTH;
next_weight <= current_weight;
next_deficit <= deficit;
end
end else begin
// 如果当前队列无效,则指针移动到下一个队列
next_pointer <= (current_pointer + 1) % DWRR_DEPTH;
next_weight <= current_weight;
next_deficit <= deficit;
end
end
end
endmodule
```
以上代码中,我们使用一个`deficit`寄存器来记录当前队列的赤字,`current_weight`寄存器来记录当前队列的权重,`round_robin_pointer`寄存器来记录当前轮到哪个队列,`current_pointer`寄存器来记录当前正在处理哪个队列,`next_pointer`寄存器来记录下一个队列,`next_weight`寄存器来记录下一个队列的权重,`next_deficit`寄存器来记录下一个队列的赤字。每个时钟周期,我们都计算出下一个队列的权重和赤字,并判断当前队列是否需要输出。如果当前队列需要输出,则更新下一个队列的权重和赤字,并将输出信号设置为有效。否则,我们只需将指针移动到下一个队列即可。
相关推荐
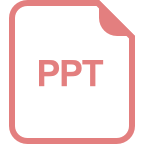




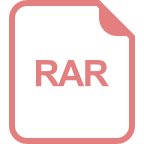
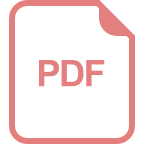
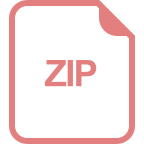
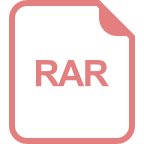