使用python爬取微博热门新闻并且输出到一个文本文档里
时间: 2024-05-01 07:16:26 浏览: 168
以下是使用Python爬取微博热门新闻并输出到文本文档的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 微博热搜的URL
url = "https://s.weibo.com/top/summary?cate=realtimehot"
# 发送HTTP请求并获取响应内容
response = requests.get(url)
html = response.text
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(html, "html.parser")
# 获取热门新闻的列表
news_list = soup.select(".td-02 a")
# 打开一个文本文件用于写入热门新闻
with open("weibo_hot_news.txt", "w", encoding="utf-8") as f:
# 遍历热门新闻列表,依次写入文件
for news in news_list:
news_title = news.get_text(strip=True)
f.write(news_title + "\n")
```
以上代码会爬取微博热搜的页面,获取热门新闻的标题,并将其写入一个名为"weibo_hot_news.txt"的文本文件中。你可以修改代码来适应你所需的输出格式。
相关问题
用python爬取微博评论区并且生成词云
在Python中,爬取微博评论并生成词云通常涉及几个步骤:
1. **网络爬虫库选择**:首先,你需要使用如`requests`库来发送HTTP请求获取网页内容,`BeautifulSoup`或`lxml`等解析库来解析HTML文档。
```python
import requests
from bs4 import BeautifulSoup
```
2. **登录与获取评论页**:如果你需要登录才能查看评论,可以使用`selenium`库配合浏览器模拟操作。如果没有登录限制,可以直接访问评论页URL。
3. **提取评论数据**:遍历解析后的HTML,找到包含评论文本的部分,这通常隐藏在JavaScript渲染后的DOM元素里,可能需要用到如`jsoup`或`pymysql`来处理动态加载的内容。
4. **数据清洗**:去掉无关字符(如标点、数字),只保留文本部分,并转换成小写。
5. **词云生成**:使用`wordcloud`库创建词云图。首先安装它:`pip install wordcloud`. 示例代码如下:
```python
from wordcloud import WordCloud
# 将所有评论合并成一个字符串
all_comments = ' '.join(cleaned_comments)
# 生成词云
wc = WordCloud(font_path='simhei.ttf', background_color='white').generate(all_comments)
wc.to_file('comments_wordcloud.png')
```
其中,`font_path`指定中文字体文件路径,`background_color`设置背景颜色。
**注意事项**:
- 微博爬虫可能会受到反爬机制保护,频繁抓取可能被封IP。请确保遵守网站的使用条款和robots.txt规则。
- 由于隐私和版权问题,直接用于个人项目前最好获得微博的授权或者只对公开评论进行分析。
使用python爬取微博近两天的前十个热搜,写入文档nove.txt,使用jieba进行词频统计,结果排序输出到result.csv中,最后生成词云图。
好的,这是一个比较复杂的任务,但是可以分步骤来完成。首先,我们需要使用Python爬取微博热搜榜单上的热门关键词。可以使用`requests`和`BeautifulSoup`库来完成这个任务。以下是代码示例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://s.weibo.com/top/summary'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
keywords = soup.select('.td-02 a')
with open('nove.txt', 'w', encoding='utf-8') as f:
for i in range(10):
keyword = keywords[i].text.strip()
f.write(keyword + '\n')
```
这段代码会将前十个热门关键词写入到`nove.txt`文件中。
接下来,我们需要使用`jieba`库进行分词和词频统计。以下是示例代码:
```python
import jieba
import csv
jieba.setLogLevel(20)
jieba.load_userdict('userdict.txt')
with open('nove.txt', 'r', encoding='utf-8') as f:
text = f.read()
words = jieba.lcut(text)
word_counts = {}
for word in words:
if len(word) == 1:
continue
elif word == '微博':
continue
else:
word_counts[word] = word_counts.get(word, 0) + 1
word_counts_items = list(word_counts.items())
word_counts_items.sort(key=lambda x: x[1], reverse=True)
with open('result.csv', 'w', encoding='utf-8-sig', newline='') as f:
writer = csv.writer(f)
writer.writerow(['词语', '词频'])
for item in word_counts_items:
writer.writerow([item[0], item[1]])
```
这段代码会将`nove.txt`中的文本进行分词和词频统计,并将结果按照词频排序写入到`result.csv`文件中。
最后,我们可以使用`wordcloud`库生成词云图。以下是示例代码:
```python
import wordcloud
with open('nove.txt', 'r', encoding='utf-8') as f:
text = f.read()
wc = wordcloud.WordCloud(
background_color='white',
font_path='C:/Windows/Fonts/simhei.ttf',
max_words=100,
width=800,
height=600,
margin=2
)
wc.generate(text)
wc.to_file('wordcloud.png')
```
这段代码会将`nove.txt`中的文本生成词云图,并将结果保存到`wordcloud.png`文件中。
以上就是完整的代码示例,可以将这些代码组合起来,完成任务。
阅读全文
相关推荐
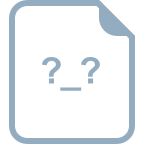
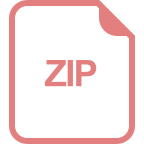
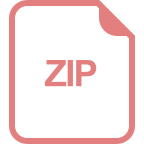
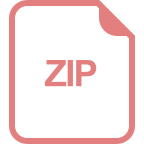
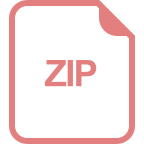
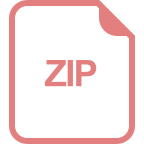

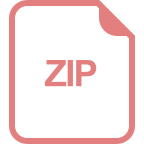
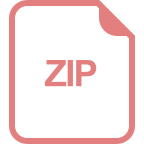
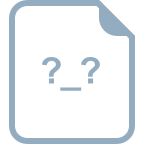
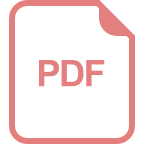
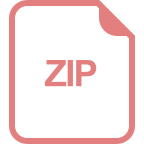
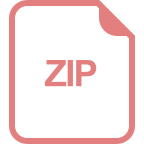


